编写一个程序,读入数目确定的考试分数,并且判断有多少个分数高于或等于平均分,有多少个分数低于平均分。输入一个负数标志输入结束,假设最高分为100。
时间: 2023-04-15 18:01:26 浏览: 89
以下是Python代码实现:
scores = [] # 用列表存储输入的分数
sum_score = 0 # 总分数
count = 0 # 分数个数
while True:
score = int(input("请输入一个分数(输入负数结束):"))
if score < 0:
break
if score > 100:
print("分数不能超过100,请重新输入!")
continue
scores.append(score)
sum_score += score
count += 1
if count == 0:
print("没有输入分数!")
else:
avg_score = sum_score / count # 平均分
above_avg = 0 # 高于或等于平均分的个数
below_avg = 0 # 低于平均分的个数
for score in scores:
if score >= avg_score:
above_avg += 1
else:
below_avg += 1
print("平均分为:", avg_score)
print("高于或等于平均分的分数个数为:", above_avg)
print("低于平均分的分数个数为:", below_avg)
相关问题
Java编写一个程序,读入数目确定的考试分数,并且判断有多少个分数高于或等于平均分,有多少个分数低于平均分。输入一个负数标志输入结束,假设最高分为100。
好的,以下是您所需的Java程序:
```java
import java.util.Scanner;
public class ExamScores {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int count = 0;
int sum = 0;
int score = 0;
int highScore = 100;
System.out.print("Enter scores (-1 to end): ");
while (score >= 0) {
score = input.nextInt();
if (score >= 0 && score <= highScore) {
sum += score;
count++;
}
}
if (count > 0) {
double average = (double) sum / count;
int aboveAverage = 0;
int belowAverage = 0;
input.close();
input = new Scanner(System.in);
System.out.print("Enter scores again (-1 to end): ");
while (score >= 0) {
score = input.nextInt();
if (score >= 0 && score <= highScore) {
if (score >= average) {
aboveAverage++;
} else {
belowAverage++;
}
}
}
System.out.println("Average score is " + average);
System.out.println("Number of scores above or equal to the average is " + aboveAverage);
System.out.println("Number of scores below the average is " + belowAverage);
} else {
System.out.println("No valid scores entered.");
}
}
}
```
这个程序使用了两个 while 循环。第一个循环用于读入分数,计算总分和分数个数,以便计算平均分数。第二个循环用于再次读入分数,并计算高于或等于平均分数的个数和低于平均分数的个数。
注意,当输入负数时,程序将退出第一个循环并开始第二个循环。如果没有有效的分数输入,则程序将输出一条相应的消息。
java编写一个程序,读入数目确定的考试分数,并且判断有多少个分数高于或等于平均分,有多少个分数低于平均分。输入一个负数标志输入结束,假设最高分为100。
以下是 Java 代码实现:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int sum = 0;
int count = 0;
int score;
int average;
while (true) {
score = scanner.nextInt();
if (score < 0) {
break;
}
sum += score;
count++;
}
average = sum / count;
int above = 0;
int below = 0;
scanner = new Scanner(System.in);
while (true) {
score = scanner.nextInt();
if (score < 0) {
break;
}
if (score >= average) {
above++;
} else {
below++;
}
}
System.out.println("平均分:" + average);
System.out.println("高于或等于平均分的人数:" + above);
System.out.println("低于平均分的人数:" + below);
}
}
希望能够帮到你!
相关推荐
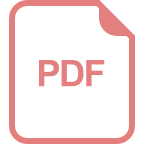
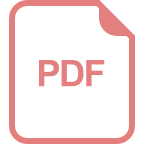
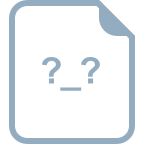












