pytorch构建CNN-GRU
时间: 2023-06-28 10:12:01 浏览: 68
首先,导入需要的库:
```python
import torch
import torch.nn as nn
```
接下来,我们定义一个CNN-GRU模型:
```python
class CNN_GRU(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(CNN_GRU, self).__init__()
# 定义CNN层
self.conv1 = nn.Conv2d(in_channels=1, out_channels=16, kernel_size=5, stride=1, padding=2)
self.pool1 = nn.MaxPool2d(kernel_size=2)
self.conv2 = nn.Conv2d(in_channels=16, out_channels=32, kernel_size=5, stride=1, padding=2)
self.pool2 = nn.MaxPool2d(kernel_size=2)
self.conv3 = nn.Conv2d(in_channels=32, out_channels=64, kernel_size=5, stride=1, padding=2)
self.pool3 = nn.MaxPool2d(kernel_size=2)
# 定义GRU层
self.gru = nn.GRU(input_size, hidden_size, batch_first=True)
# 定义全连接层
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, x):
# CNN层
x = self.conv1(x)
x = torch.relu(x)
x = self.pool1(x)
x = self.conv2(x)
x = torch.relu(x)
x = self.pool2(x)
x = self.conv3(x)
x = torch.relu(x)
x = self.pool3(x)
# 转换输入形状以适用于GRU层
x = x.permute(0, 2, 1, 3) # (batch_size, height, width, channels) -> (batch_size, width, height, channels)
x = x.reshape(x.shape[0], x.shape[1], -1) # (batch_size, width, height * channels)
# GRU层
x, _ = self.gru(x)
# 全连接层
x = self.fc(x[:, -1, :])
x = torch.sigmoid(x)
return x
```
在这个模型中,我们首先定义了一个CNN层,用于从输入的图像中提取特征。然后,我们将输出的特征序列通过GRU层,最后通过一个全连接层,将输出映射到指定的输出维度。
在`forward`方法中,我们首先将输入通过CNN层,并将输出的形状转换为适用于GRU层的形状。然后,我们将x传递给GRU层,并取出GRU层的最后一个输出。最后,我们将GRU层的最后一个输出通过全连接层,并使用sigmoid激活函数输出预测结果。
最后,我们可以根据需要的输入、隐藏和输出大小来实例化一个CNN-GRU模型:
```python
model = CNN_GRU(input_size=64, hidden_size=128, output_size=10)
```
阅读全文
相关推荐
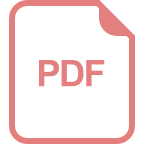



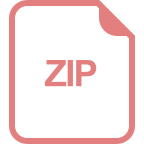
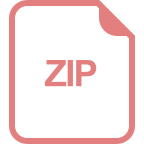
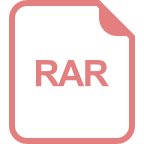
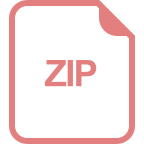
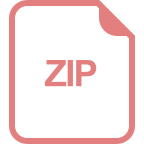
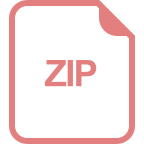
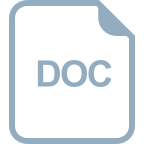
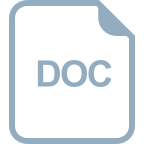
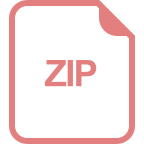
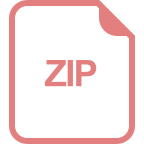
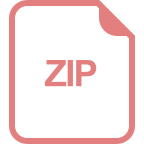
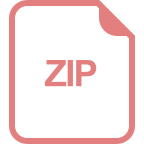
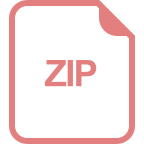