随机生成一个普通方法java
时间: 2023-08-04 19:37:56 浏览: 41
好的,这里是一个随机生成指定长度字符串的普通方法Java示例:
```java
import java.util.Random;
public class StringUtil {
public static String generateRandomString(int length) {
String chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
StringBuilder sb = new StringBuilder(length);
Random random = new Random();
for (int i = 0; i < length; i++) {
sb.append(chars.charAt(random.nextInt(chars.length())));
}
return sb.toString();
}
}
```
这个方法接收一个整数参数 `length`,返回一个由随机生成的大写字母、小写字母和数字组成的字符串,长度为 `length`。可以根据需要进行修改和扩展。
相关问题
java实现认证随机生成二进制数
你可以使用Java中的SecureRandom类来生成认证随机的二进制数。下面是一个示例代码:
```java
import java.security.SecureRandom;
public class AuthenticationGenerator {
public static void main(String[] args) {
int bitLength = 256; // 设置生成的二进制数的位数
byte[] randomBytes = generateRandomBytes(bitLength);
String binaryString = bytesToBinaryString(randomBytes);
System.out.println("随机生成的二进制数:" + binaryString);
}
private static byte[] generateRandomBytes(int bitLength) {
int byteLength = bitLength / 8; // 计算生成的随机字节数
SecureRandom secureRandom = new SecureRandom();
byte[] randomBytes = new byte[byteLength];
secureRandom.nextBytes(randomBytes); // 生成随机字节数组
return randomBytes;
}
private static String bytesToBinaryString(byte[] bytes) {
StringBuilder binaryString = new StringBuilder();
for (byte b : bytes) {
for (int i = 7; i >= 0; i--) {
binaryString.append((b >> i) & 1); // 将每个字节转换为二进制字符串
}
}
return binaryString.toString();
}
}
```
上述代码中,我们通过调用SecureRandom类的nextBytes方法生成指定位数的随机字节数组。然后,我们将每个字节转换为二进制字符串,并将它们连接起来形成最终的二进制数。
请注意,这里使用的是SecureRandom类而不是普通的Random类,因为SecureRandom提供了更强的随机性和安全性。
用随机投点法计算圆周率pi值的java代码
下面是用随机投点法计算圆周率pi值的Java代码:
```java
public class CalculatePi {
public static void main(String[] args) {
int n = 1000000; // 随机投点的次数
int inside = 0; // 落在圆内的点数
for (int i = 0; i < n; i++) {
double x = Math.random(); // 生成x坐标
double y = Math.random(); // 生成y坐标
if (x * x + y * y <= 1) { // 判断是否在圆内
inside++;
}
}
double pi = 4.0 * inside / n; // 计算pi值
System.out.println("pi = " + pi);
}
}
```
该代码通过随机生成点的坐标,判断这些点是否在圆内,最后根据落在圆内的点数和总点数计算圆周率pi值。
相关推荐
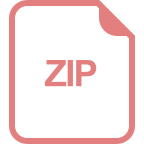
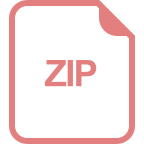












