进程间的通信系统代码实现界面python
时间: 2023-07-25 16:18:53 浏览: 130
以下是一个使用Python实现的进程间通信的简单界面,包括了管道通信和消息队列通信的示例代码。
```python
import tkinter as tk
import os
import time
import threading
import queue
import sysv_ipc
# 定义消息队列的消息结构
class MsgBuf(ctypes.Structure):
_fields_ = [("mtype", ctypes.c_long), ("mtext", ctypes.c_char * 1024)]
# 创建消息队列
msgq_key = 0x1234
msgq = sysv_ipc.MessageQueue(msgq_key, sysv_ipc.IPC_CREAT)
# 定义管道的读写文件描述符
r_fd, w_fd = os.pipe()
# 定义全局变量
msg = ''
pipe_msg = ''
# 定义一个队列,用于从线程中获取消息
msg_queue = queue.Queue()
# 定义线程,用于接收管道消息
def pipe_thread():
global pipe_msg
while True:
pipe_msg = os.read(r_fd, 1024).decode()
msg_queue.put('pipe')
# 定义线程,用于接收消息队列消息
def msgq_thread():
global msg
while True:
msg_buf, msg_type = msgq.receive()
msg = msg_buf.decode()
msg_queue.put('msgq')
# 启动线程
t1 = threading.Thread(target=pipe_thread)
t1.setDaemon(True)
t1.start()
t2 = threading.Thread(target=msgq_thread)
t2.setDaemon(True)
t2.start()
# 定义GUI界面
class App:
def __init__(self, master):
self.master = master
master.title("进程间通信示例")
# 管道通信示例
self.pipe_label = tk.Label(master, text="管道通信示例")
self.pipe_label.grid(row=0, column=0)
self.pipe_send_button = tk.Button(master, text="发送消息", command=self.pipe_send)
self.pipe_send_button.grid(row=1, column=0)
self.pipe_recv_label = tk.Label(master, text="接收到的消息:")
self.pipe_recv_label.grid(row=2, column=0)
self.pipe_recv_text = tk.Text(master, height=1, width=30)
self.pipe_recv_text.grid(row=3, column=0)
# 消息队列通信示例
self.msgq_label = tk.Label(master, text="消息队列通信示例")
self.msgq_label.grid(row=0, column=1)
self.msgq_send_button = tk.Button(master, text="发送消息", command=self.msgq_send)
self.msgq_send_button.grid(row=1, column=1)
self.msgq_recv_label = tk.Label(master, text="接收到的消息:")
self.msgq_recv_label.grid(row=2, column=1)
self.msgq_recv_text = tk.Text(master, height=1, width=30)
self.msgq_recv_text.grid(row=3, column=1)
# 定时更新界面
self.update_clock()
# 发送管道消息
def pipe_send(self):
msg = "Hello, pipe!"
os.write(w_fd, msg.encode())
# 发送消息队列消息
def msgq_send(self):
msg = "Hello, msgq!"
msgq.send(msg.encode(), True)
# 更新界面
def update_clock(self):
try:
msg_type = msg_queue.get_nowait()
if msg_type == 'pipe':
self.pipe_recv_text.delete(1.0, tk.END)
self.pipe_recv_text.insert(tk.END, pipe_msg)
elif msg_type == 'msgq':
self.msgq_recv_text.delete(1.0, tk.END)
self.msgq_recv_text.insert(tk.END, msg)
except queue.Empty:
pass
self.master.after(100, self.update_clock)
# 启动GUI界面
root = tk.Tk()
app = App(root)
root.mainloop()
```
以上代码中,使用了Python的Tkinter库实现了一个简单的GUI界面,包括了管道通信和消息队列通信的示例代码。在GUI界面中,用户可以点击“发送消息”按钮向管道或者消息队列发送消息,并且实时显示接收到的消息。程序中使用了两个线程分别用于接收管道消息和消息队列消息,消息接收后通过一个队列传递给主线程进行更新界面。
阅读全文
相关推荐
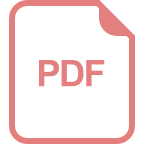
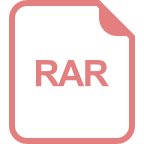
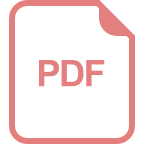
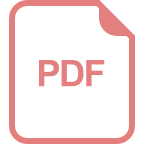
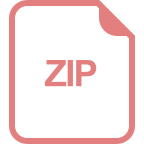
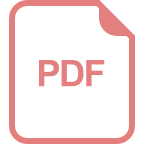
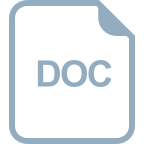
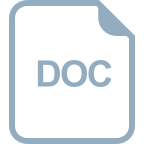
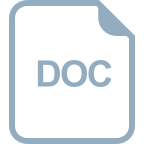
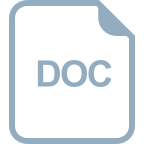
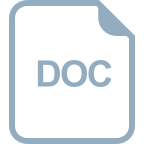
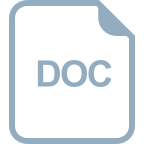
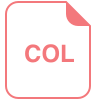
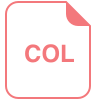
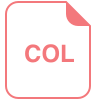
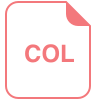
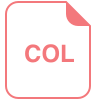
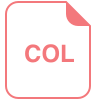