使用Java代码从后端获取用户列表并动态添加到表格中
时间: 2024-04-09 22:29:56 浏览: 24
要从后端获取用户列表并将其动态添加到表格中,你可以使用Java代码和HTML结合的方式进行实现。以下是一个简单的示例:
首先,创建一个Java Servlet来处理后端请求和响应。在这个Servlet中,你需要从后端获取用户列表的数据,并将其存储在适当的数据结构中(比如List、数组等)。
```java
@WebServlet("/userList")
public class UserListServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 从后端获取用户列表数据
List<User> userList = UserService.getUserList(); // 假设UserService是一个获取用户列表的服务类
// 将用户列表数据存储在request属性中
request.setAttribute("userList", userList);
// 转发请求到JSP页面
request.getRequestDispatcher("userList.jsp").forward(request, response);
}
}
```
接下来,在JSP页面中,你可以使用JSTL标签库和EL表达式来动态地将用户列表添加到表格中。
```jsp
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<title>User List</title>
</head>
<body>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<c:forEach var="user" items="${userList}">
<tr>
<td>${user.id}</td>
<td>${user.name}</td>
<td>${user.email}</td>
</tr>
</c:forEach>
</tbody>
</table>
</body>
</html>
```
在上述代码中,使用`c:forEach`标签遍历`${userList}`中的每个用户对象,并将其属性值动态地添加到表格的每一行。
最后,你需要在web.xml文件中配置Servlet的映射:
```xml
<servlet>
<servlet-name>UserListServlet</servlet-name>
<servlet-class>com.example.UserListServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>UserListServlet</servlet-name>
<url-pattern>/userList</url-pattern>
</servlet-mapping>
```
这样,当访问`/userList`路径时,会触发UserListServlet的doGet方法,从后端获取用户列表并将其传递给userList.jsp页面进行渲染。
请注意,上述代码仅为示例,实际中你需要根据你的数据结构和业务逻辑进行适当的修改。
相关推荐
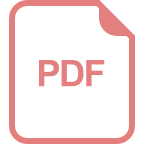














