xdp程序如何将数据包从一个接口转发到另一个接口,请给一个完整的示例
时间: 2024-05-05 18:15:06 浏览: 300
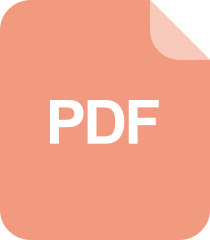
数据包转发实例1
以下是一个简单的示例,演示如何使用XDP程序将数据包从一个接口转发到另一个接口:
1. 首先,定义一个XDP程序,它将捕获传入的数据包并将其转发到指定的接口。以下是一个示例程序:
```c
#include <linux/bpf.h>
#include <linux/if_ether.h>
#include <linux/ip.h>
#include <linux/in.h>
SEC("xdp")
int xdp_prog(struct xdp_md *ctx)
{
void *data_end = (void *)(long)ctx->data_end;
void *data = (void *)(long)ctx->data;
struct ethhdr *eth = data;
struct iphdr *ip = data + sizeof(*eth);
// Check if packet is IPv4
if (eth->h_proto != htons(ETH_P_IP)) {
return XDP_PASS;
}
// Set destination MAC to target interface MAC
eth->h_dest[0] = 0x00;
eth->h_dest[1] = 0x11;
eth->h_dest[2] = 0x22;
eth->h_dest[3] = 0x33;
eth->h_dest[4] = 0x44;
eth->h_dest[5] = 0x55;
// Set source MAC to current interface MAC
eth->h_source[0] = 0x66;
eth->h_source[1] = 0x77;
eth->h_source[2] = 0x88;
eth->h_source[3] = 0x99;
eth->h_source[4] = 0xAA;
eth->h_source[5] = 0xBB;
// Update IP checksum
ip->check = 0;
ip->check = ~csum_fold(csum_partial(ip, sizeof(*ip), 0));
// Forward packet to target interface
return bpf_redirect_map(&forward_map, 0, BPF_F_NOEXIST);
}
```
2. 接下来,我们需要创建一个哈希映射,将目标接口的索引映射到对应的输出队列。以下是一个示例代码:
```c
struct bpf_map_def SEC("maps") forward_map = {
.type = BPF_MAP_TYPE_DEVMAP_HASH,
.key_size = sizeof(int),
.value_size = sizeof(int),
.max_entries = 64,
};
```
在此示例中,我们使用`BPF_MAP_TYPE_DEVMAP_HASH`类型的哈希映射,将目标接口的索引映射到输出队列的索引。`key_size`和`value_size`参数分别指定键和值的大小,`max_entries`参数指定哈希表的最大条目数。
3. 最后,我们需要将XDP程序附加到目标接口。以下是示例代码:
```c
int ifindex = if_nametoindex("eth1");
if (ifindex == 0) {
fprintf(stderr, "Interface not found\n");
return 1;
}
int prog_fd = bpf_program__fd(prog);
if (bpf_set_link_xdp_fd(ifindex, prog_fd, 0) == -1) {
fprintf(stderr, "Failed to attach XDP program\n");
return 1;
}
```
在此示例中,我们使用`if_nametoindex`函数查找目标接口的索引,然后使用`bpf_set_link_xdp_fd`函数将XDP程序附加到该接口。`prog_fd`参数是XDP程序的文件描述符,`0`参数指定程序应该附加到RX队列。
完成以上步骤后,XDP程序将能够捕获传入的数据包,并将其转发到目标接口。请注意,我们在示例程序中硬编码了目标接口的MAC地址和IP地址。在实际使用中,您需要动态获取这些信息,例如从ARP缓存中读取目标MAC地址。
阅读全文
相关推荐

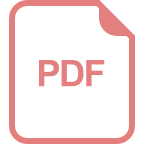
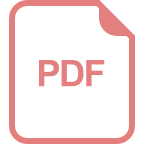
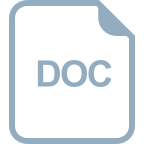
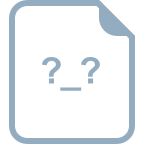
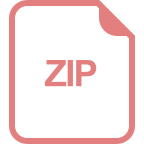
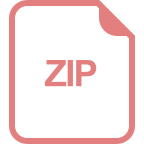
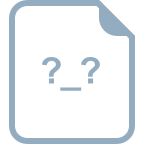
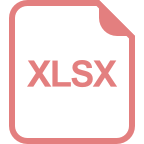
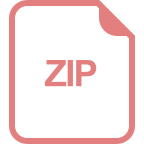
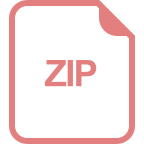