xdp程序如何将一个接口收到的数据包转发到另一个接口,请给一个完整的例子
时间: 2024-04-30 17:24:06 浏览: 274
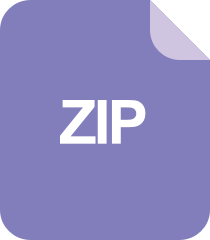
像XDP数据包捕获一样的tcpdump-Golang开发
以下是一个基本的XDP程序示例,将接收到的数据包从一个接口转发到另一个接口。
该程序使用了libbpf和libpcap库来捕获和发送数据包。
```c
#include <linux/bpf.h>
#include <linux/if_ether.h>
#include <linux/ip.h>
#include <linux/in.h>
#include <linux/tcp.h>
#include <linux/udp.h>
#include <linux/filter.h>
#include <linux/if_packet.h>
#include <linux/if_vlan.h>
#include <linux/if_xdp.h>
#include <arpa/inet.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <unistd.h>
#include <net/if.h>
#include <pcap.h>
#include <bpf/bpf.h>
#include <bpf/libbpf.h>
#define MAX_PACKET_SIZE 2048
static char *iface_in = "eth0"; // 接收数据包的接口
static char *iface_out = "eth1"; // 转发数据包的接口
struct bpf_map_def SEC("maps") xdp_stats_map = {
.type = BPF_MAP_TYPE_ARRAY,
.key_size = sizeof(__u32),
.value_size = sizeof(__u64),
.max_entries = XDP_ACTION_MAX,
};
// XDP程序函数
SEC("xdp_prog")
int xdp_prog(struct xdp_md *ctx) {
void *data_end = (void *)(long)ctx->data_end;
void *data = (void *)(long)ctx->data;
__u32 action = XDP_DROP;
__u32 key = XDP_PASS;
__u64 *value;
// 解析数据包头部
struct ethhdr *eth = data;
if (eth + 1 > (struct ethhdr *)data_end) {
return XDP_DROP;
}
// 判断是否为IP数据包
if (ntohs(eth->h_proto) == ETH_P_IP) {
struct iphdr *ip = data + sizeof(*eth);
if (ip + 1 > (struct iphdr *)data_end) {
return XDP_DROP;
}
// 判断是否为TCP或UDP数据包
if (ip->protocol == IPPROTO_TCP || ip->protocol == IPPROTO_UDP) {
struct tcphdr *tcp = data + sizeof(*eth) + sizeof(*ip);
struct udphdr *udp = data + sizeof(*eth) + sizeof(*ip);
if (ip->protocol == IPPROTO_TCP && tcp + 1 > (struct tcphdr *)data_end) {
return XDP_DROP;
}
if (ip->protocol == IPPROTO_UDP && udp + 1 > (struct udphdr *)data_end) {
return XDP_DROP;
}
// 将数据包转发到另一个接口
struct bpf_sock_tuple tuple = {
.src_ip4 = ip->saddr,
.dst_ip4 = ip->daddr,
.src_port = tcp ? tcp->source : udp->source,
.dst_port = tcp ? tcp->dest : udp->dest,
.family = AF_INET,
.protocol = ip->protocol,
};
action = bpf_redirect_map(&xdp_stats_map, key, 0, &tuple, sizeof(tuple));
}
}
value = bpf_map_lookup_elem(&xdp_stats_map, &action);
if (value) {
*value += 1;
}
return action;
}
int main(int argc, char **argv) {
int err, in_ifindex, out_ifindex, prog_fd;
struct bpf_object *obj;
struct bpf_program *prog;
struct bpf_map *map;
pcap_t *pcap;
char errbuf[PCAP_ERRBUF_SIZE];
struct bpf_prog_load_attr prog_load_attr = {
.prog_type = BPF_PROG_TYPE_XDP,
.expected_attach_type = BPF_XDP_IN,
};
// 获取接口的ifindex
in_ifindex = if_nametoindex(iface_in);
if (!in_ifindex) {
fprintf(stderr, "Invalid input interface: %s\n", iface_in);
return 1;
}
out_ifindex = if_nametoindex(iface_out);
if (!out_ifindex) {
fprintf(stderr, "Invalid output interface: %s\n", iface_out);
return 1;
}
// 加载XDP程序
err = bpf_prog_load("xdp_prog.o", BPF_PROG_TYPE_XDP, &obj, &prog_fd);
if (err) {
fprintf(stderr, "Failed to load XDP program: %s\n", strerror(errno));
return 1;
}
// 获取XDP程序
prog = bpf_object__find_program_by_title(obj, "xdp_prog");
if (!prog) {
fprintf(stderr, "Failed to find XDP program\n");
return 1;
}
// 将XDP程序附加到接口上
err = bpf_set_link_xdp_fd(in_ifindex, prog_fd, 0);
if (err) {
fprintf(stderr, "Failed to attach XDP program to interface: %s\n", strerror(errno));
return 1;
}
// 创建map
map = bpf_object__find_map_by_name(obj, "xdp_stats_map");
if (!map) {
fprintf(stderr, "Failed to find map: xdp_stats_map\n");
return 1;
}
// 打开pcap
pcap = pcap_open_live(iface_out, MAX_PACKET_SIZE, 1, 1000, errbuf);
if (!pcap) {
fprintf(stderr, "Failed to open pcap: %s\n", errbuf);
return 1;
}
// 循环捕获数据包并转发
for (;;) {
struct pcap_pkthdr *hdr;
const u_char *packet;
err = pcap_next_ex(pcap, &hdr, &packet);
if (err <= 0) {
continue;
}
// 发送数据包到另一个接口
err = bpf_send_helper(map, out_ifindex, packet, hdr->caplen);
if (err < 0) {
fprintf(stderr, "Failed to send packet: %s\n", strerror(errno));
return 1;
}
}
// 将XDP程序从接口上移除
bpf_set_link_xdp_fd(in_ifindex, -1, 0);
return 0;
}
```
该示例程序将接收到的数据包从eth0接口转发到eth1接口。在XDP程序中,它会检查数据包是否为IP、TCP或UDP数据包,如果是,则将其转发到另一个接口。转发使用了bpf_redirect_map函数,它会将数据包发送到map中指定的接口。在本例中,map指定为xdp_stats_map。
在主函数中,程序使用libpcap库来捕获eth1接口上的数据包,并使用bpf_send_helper函数将它们发送到eth0接口上。
请注意,此示例程序仅用于演示目的。实际使用时,您需要对其进行适当修改以满足您的需求,并根据您的环境进行测试和验证。
阅读全文
相关推荐
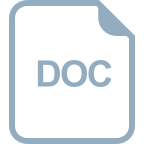
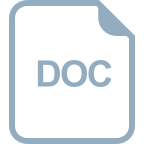


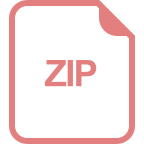
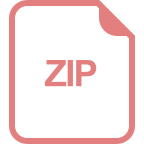
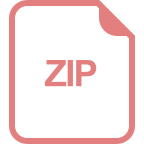










