1.自定义一个class Computer,有公有数据成员 m_price 和 m_vendor, 分别表示电脑的价格和生产商;要求重载输出运算符 << 和 比较运算符< (按价格大小比较) 2.主程序main()里,定义4台电脑:("Lenovo", 7800.0) ("Dell", 8000.0) ("Huashuo", 6000.0) ("Apple", 10000.0) 把电脑加入到随机访问容器中,然后使用sort()算法,分别实现按价格排序(调用Computer对象比较运算符<)和结果输出,以及按生产商(使用lambda表达式)排序和输出。 输入格式: 无 输出格式: 电脑信息输出:生产商在前价格在后,中间空格隔开 排序结果:每一行输出一个电脑信息
时间: 2024-03-06 19:50:18 浏览: 97
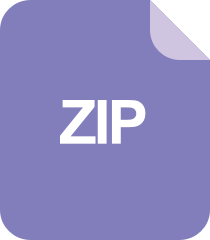
usb device(vid_1f3a_pid_efe8)
好的,我来回答你的问题。
以下是对应的C++代码:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
#include <string>
#include <ctime>
using namespace std;
class Computer {
public:
Computer(string vendor = "", double price = 0.0) : m_vendor(vendor), m_price(price) {}
friend ostream& operator<<(ostream& os, const Computer& c) {
os << c.m_vendor << " " << c.m_price;
return os;
}
bool operator<(const Computer& c) const {
return m_price < c.m_price;
}
private:
string m_vendor;
double m_price;
};
int main() {
srand(time(nullptr));
vector<Computer> computers;
computers.emplace_back("Lenovo", 7800.0);
computers.emplace_back("Dell", 8000.0);
computers.emplace_back("Huashuo", 6000.0);
computers.emplace_back("Apple", 10000.0);
random_shuffle(computers.begin(), computers.end());
cout << "按价格排序:" << endl;
sort(computers.begin(), computers.end());
for (const auto& c : computers) {
cout << c << endl;
}
cout << endl << "按生产商排序:" << endl;
sort(computers.begin(), computers.end(), [](const Computer& c1, const Computer& c2) {
return c1.m_vendor < c2.m_vendor;
});
for (const auto& c : computers) {
cout << c << endl;
}
return 0;
}
```
顺便说一下,这里我用了C++11的一些新特性,比如随机打乱容器元素的函数 `random_shuffle`,以及 lambda 表达式。
阅读全文
相关推荐
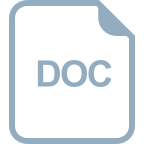
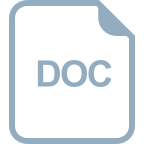
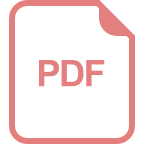
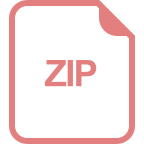
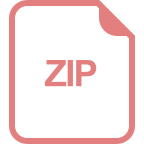
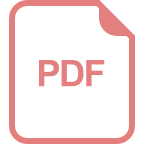
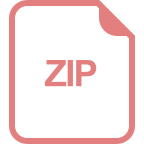
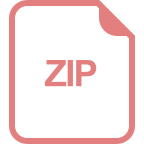
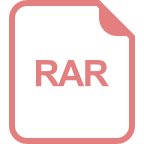
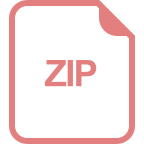
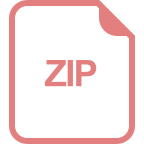
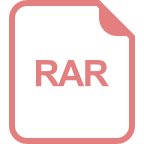
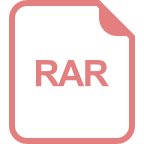
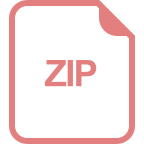
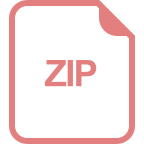
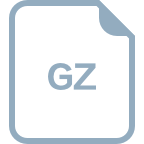