Java:分别利用 synchronized、Lock和阻塞队列的方法模拟生产者-消费者的同步问题。 分别利用 synchronized、Lock和阻塞队列的方法实现交替输出ABABABAB。
时间: 2024-03-14 18:44:32 浏览: 65
生产者-消费者同步问题的模拟:
使用synchronized:
```java
public class ProducerConsumer {
private static final int BUFFER_SIZE = 10;
private static final Object lock = new Object();
private static int[] buffer = new int[BUFFER_SIZE];
private static int count = 0;
public static void main(String[] args) {
Thread producer = new Thread(() -> {
for (int i = 0; i < BUFFER_SIZE; i++) {
synchronized (lock) {
while (count == BUFFER_SIZE) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
buffer[count++] = i;
lock.notifyAll();
}
}
});
Thread consumer = new Thread(() -> {
for (int i = 0; i < BUFFER_SIZE; i++) {
synchronized (lock) {
while (count == 0) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println(buffer[--count]);
lock.notifyAll();
}
}
});
producer.start();
consumer.start();
}
}
```
使用Lock:
```java
public class ProducerConsumer {
private static final int BUFFER_SIZE = 10;
private static final Lock lock = new ReentrantLock();
private static final Condition notFull = lock.newCondition();
private static final Condition notEmpty = lock.newCondition();
private static int[] buffer = new int[BUFFER_SIZE];
private static int count = 0;
public static void main(String[] args) {
Thread producer = new Thread(() -> {
for (int i = 0; i < BUFFER_SIZE; i++) {
lock.lock();
try {
while (count == BUFFER_SIZE) {
notFull.await();
}
buffer[count++] = i;
notEmpty.signalAll();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
});
Thread consumer = new Thread(() -> {
for (int i = 0; i < BUFFER_SIZE; i++) {
lock.lock();
try {
while (count == 0) {
notEmpty.await();
}
System.out.println(buffer[--count]);
notFull.signalAll();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
});
producer.start();
consumer.start();
}
}
```
使用阻塞队列:
```java
public class ProducerConsumer {
private static final int BUFFER_SIZE = 10;
public static void main(String[] args) {
BlockingQueue<Integer> buffer = new ArrayBlockingQueue<>(BUFFER_SIZE);
Thread producer = new Thread(() -> {
for (int i = 0; i < BUFFER_SIZE; i++) {
try {
buffer.put(i);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
});
Thread consumer = new Thread(() -> {
for (int i = 0; i < BUFFER_SIZE; i++) {
try {
System.out.println(buffer.take());
} catch (InterruptedException e) {
e.printStackTrace();
}
}
});
producer.start();
consumer.start();
}
}
```
交替输出ABABABAB的实现:
使用synchronized:
```java
public class AlternatePrinting {
private static final Object lock = new Object();
private static boolean flag = true;
public static void main(String[] args) {
Thread t1 = new Thread(() -> {
for (int i = 0; i < 5; i++) {
synchronized (lock) {
while (!flag) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.print("A");
flag = false;
lock.notifyAll();
}
}
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 5; i++) {
synchronized (lock) {
while (flag) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.print("B");
flag = true;
lock.notifyAll();
}
}
});
t1.start();
t2.start();
}
}
```
使用Lock:
```java
public class AlternatePrinting {
private static final Lock lock = new ReentrantLock();
private static final Condition condition = lock.newCondition();
private static boolean flag = true;
public static void main(String[] args) {
Thread t1 = new Thread(() -> {
for (int i = 0; i < 5; i++) {
lock.lock();
try {
while (!flag) {
condition.await();
}
System.out.print("A");
flag = false;
condition.signalAll();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 5; i++) {
lock.lock();
try {
while (flag) {
condition.await();
}
System.out.print("B");
flag = true;
condition.signalAll();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
});
t1.start();
t2.start();
}
}
```
使用Semaphore:
```java
public class AlternatePrinting {
private static final Semaphore s1 = new Semaphore(1);
private static final Semaphore s2 = new Semaphore(0);
public static void main(String[] args) {
Thread t1 = new Thread(() -> {
for (int i = 0; i < 5; i++) {
try {
s1.acquire();
System.out.print("A");
s2.release();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 5; i++) {
try {
s2.acquire();
System.out.print("B");
s1.release();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
});
t1.start();
t2.start();
}
}
```
阅读全文
相关推荐
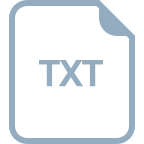
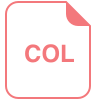
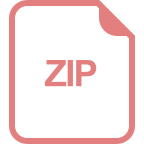
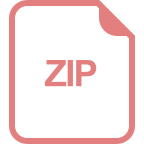
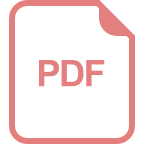
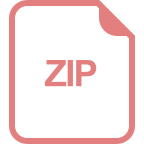
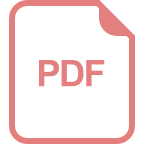
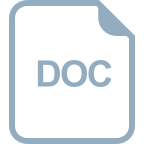
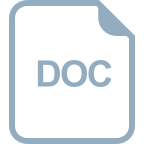
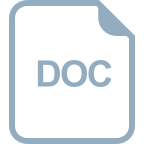
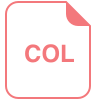
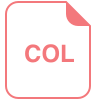
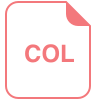
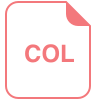
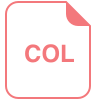
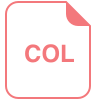