用python 写一个俄罗斯方块的小游戏
时间: 2023-08-04 21:39:20 浏览: 98
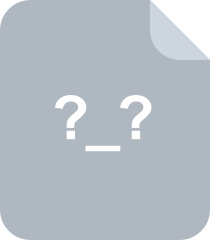
一个用python编写的俄罗斯方块小游戏.py
好的,下面是一个简单的俄罗斯方块小游戏的Python代码示例,基于Pygame库:
```python
import pygame
import random
pygame.init()
# 游戏窗口大小
WINDOW_WIDTH = 400
WINDOW_HEIGHT = 600
# 方块大小
BLOCK_SIZE = 20
# 游戏区域大小
PLAY_WIDTH = 10 * BLOCK_SIZE
PLAY_HEIGHT = 20 * BLOCK_SIZE
# 游戏区域位置
PLAY_X = (WINDOW_WIDTH - PLAY_WIDTH) // 2
PLAY_Y = WINDOW_HEIGHT - PLAY_HEIGHT - 50
# 方块类型和颜色
SHAPES = [
([(0, 0), (1, 0), (2, 0), (3, 0)], (255, 0, 0)), # I
([(0, 0), (1, 0), (0, 1), (1, 1)], (0, 255, 0)), # O
([(0, 0), (1, 0), (1, 1), (2, 1)], (0, 0, 255)), # J
([(2, 0), (1, 0), (1, 1), (0, 1)], (255, 255, 0)), # L
([(1, 0), (0, 1), (1, 1), (2, 1)], (255, 0, 255)), # T
([(0, 1), (1, 1), (1, 0), (2, 0)], (0, 255, 255)), # Z
([(2, 1), (1, 1), (1, 0), (0, 0)], (255, 128, 0)), # S
]
# 初始化游戏窗口
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption("俄罗斯方块")
# 游戏区域背景颜色
PLAY_BG_COLOR = (0, 0, 0)
# 方块移动速度
FPS = 60
clock = pygame.time.Clock()
class Block:
def __init__(self, shape, color):
self.shape = shape
self.color = color
self.x = PLAY_X + PLAY_WIDTH // 2 - BLOCK_SIZE * 2
self.y = PLAY_Y - BLOCK_SIZE * 4
# 旋转方块
def rotate(self):
self.shape = [(y, -x) for x, y in self.shape[::-1]]
# 移动方块
def move(self, dx, dy):
self.x += dx * BLOCK_SIZE
self.y += dy * BLOCK_SIZE
# 在游戏区域中检查方块是否合法
def check_valid(self, board):
for x, y in self.shape:
if not (0 <= self.x + x * BLOCK_SIZE < PLAY_WIDTH and 0 <= self.y + y * BLOCK_SIZE < PLAY_HEIGHT) or board[
(self.y + y * BLOCK_SIZE) // BLOCK_SIZE][(self.x + x * BLOCK_SIZE) // BLOCK_SIZE]:
return False
return True
# 在游戏区域中放置方块
def place(self, board):
for x, y in self.shape:
board[(self.y + y * BLOCK_SIZE) // BLOCK_SIZE][(self.x + x * BLOCK_SIZE) // BLOCK_SIZE] = self.color
# 绘制方块
def draw(self, surface):
for x, y in self.shape:
pygame.draw.rect(surface, self.color, (self.x + x * BLOCK_SIZE, self.y + y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 初始化游戏区域
board = [[(0, 0, 0) for _ in range(PLAY_WIDTH // BLOCK_SIZE)] for _ in range(PLAY_HEIGHT // BLOCK_SIZE)]
# 初始化当前方块和下一个方块
current_block = Block(*random.choice(SHAPES))
next_block = Block(*random.choice(SHAPES))
# 初始化游戏分数
score = 0
# 游戏循环
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_ESCAPE:
pygame.quit()
exit()
elif event.key == pygame.K_LEFT:
current_block.move(-1, 0)
if not current_block.check_valid(board):
current_block.move(1, 0)
elif event.key == pygame.K_RIGHT:
current_block.move(1, 0)
if not current_block.check_valid(board):
current_block.move(-1, 0)
elif event.key == pygame.K_DOWN:
current_block.move(0, 1)
if not current_block.check_valid(board):
current_block.move(0, -1)
elif event.key == pygame.K_UP:
current_block.rotate()
if not current_block.check_valid(board):
current_block.rotate()
# 更新方块位置
current_block.move(0, 1)
if not current_block.check_valid(board):
current_block.move(0, -1)
current_block.place(board)
current_block = next_block
next_block = Block(*random.choice(SHAPES))
# 消除满行
for y in range(len(board)):
if all(board[y]):
board.pop(y)
board.insert(0, [(0, 0, 0) for _ in range(PLAY_WIDTH // BLOCK_SIZE)])
score += 10
# 绘制游戏界面
window.fill(PLAY_BG_COLOR)
for y, row in enumerate(board):
for x, color in enumerate(row):
pygame.draw.rect(window, color, (PLAY_X + x * BLOCK_SIZE, PLAY_Y + y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
current_block.draw(window)
# 绘制分数
font = pygame.font.Font(None, 36)
text = font.render("Score: " + str(score), True, (255, 255, 255))
window.blit(text, (10, 10))
# 绘制下一个方块
font = pygame.font.Font(None, 24)
text = font.render("Next:", True, (255, 255, 255))
window.blit(text, (PLAY_X + PLAY_WIDTH + 20, PLAY_Y))
next_block.draw(window)
pygame.display.update()
# 控制游戏帧率
clock.tick(FPS)
```
该代码实现了一个简单的俄罗斯方块小游戏,包括方块的移动、旋转、落下、消除满行等基本操作,还有分数统计和下一个方块的预览功能。可以通过改变游戏窗口大小、方块大小、方块类型和颜色、游戏区域大小等参数来进行自定义修改。
阅读全文
相关推荐





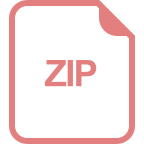
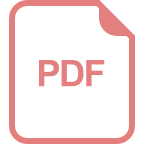
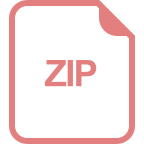
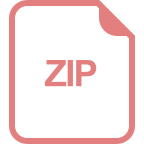
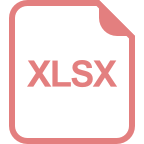
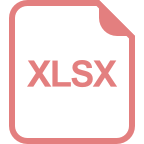