树莓派四B串口的读取函数C语言
时间: 2024-10-10 21:04:09 浏览: 34
在C语言中,如果你想从树莓派(Raspberry Pi) 4B的串口读取数据,通常会使用POSIX标准库中的`stdio.h`和`termios.h`头文件以及相关的函数。以下是一个简单的示例,展示了如何打开串口、设置波特率并读取数据:
```c
#include <stdio.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <termios.h>
#define SERIAL_PORT "/dev/ttyAMA0" // 根据实际串口号替换
#define BAUD_RATE B9600
int main() {
int fd;
struct termios options;
// 打开串口
fd = open(SERIAL_PORT, O_RDWR | O_NOCTTY | O_NDELAY); // O_RDWR用于读写,O_NOCTTY忽略控制台输入,O_NDELAY消除延迟
if (fd == -1) {
perror("Error opening serial port");
return 1;
}
// 设置串口模式
tcgetattr(fd, &options);
cfsetispeed(&options, BAUD_RATE); // 设置波特率
cfsetospeed(&options, BAUD_RATE);
options.c_cflag &= ~CSIZE; // 清除数据位大小标志,通常设置为8位
options.c_cflag |= CS8;
options.c_iflag &= ~(IGNBRK | BRKINT | PARMRK | ISTRIP); // 清除无意义标志
options.c_oflag &= ~OPOST; // 关闭发送延时
options.c_lflag &= ~(ICANON | ECHO | ISIG); // 清除终端处理标志
tcflush(fd, TCIFLUSH); // 清空接收缓冲区
tcsetattr(fd, TCSANOW, &options);
char input[256]; // 存储读取的数据
ssize_t bytes_read = read(fd, input, sizeof(input));
if (bytes_read > 0) {
printf("Read %zd bytes: %s\n", bytes_read, input);
} else {
perror("Error reading from serial port");
}
close(fd);
return 0;
}
```
这个例子假设串口设备是/dev/ttyAMA0,并且设置了9600波特率。执行程序时,它会持续监听串口输入直到有数据可用。
阅读全文
相关推荐
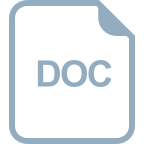
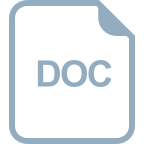
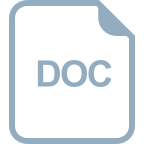
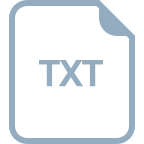
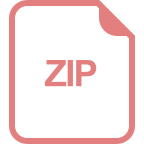
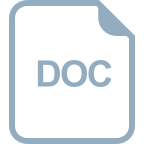
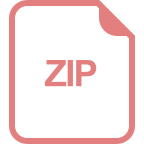
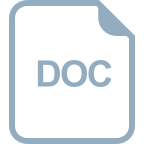

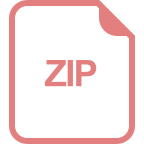
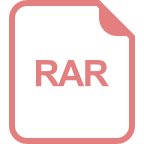
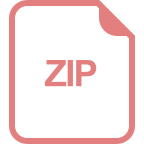
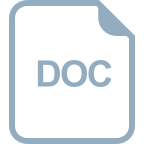
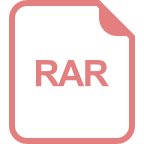
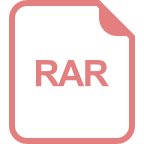
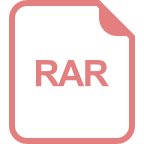
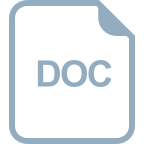
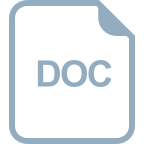
