BIO,NIO,AIO用代码举例说明
时间: 2024-06-06 18:10:00 浏览: 100
好的,下面是关于BIO、NIO、AIO的代码示例:
BIO (Blocking IO) 的示例代码:
Socket socket = new Socket("localhost", 8080);
InputStream inputStream = socket.getInputStream();
OutputStream outputStream = socket.getOutputStream();
byte[] request = "Hello, World!".getBytes();
outputStream.write(request);
byte[] response = new byte[1024];
int read = inputStream.read(response);
byte[] result = Arrays.copyOf(response, read);
System.out.println(new String(result));
NIO (Non-Blocking IO) 的示例代码:
SocketChannel socketChannel = SocketChannel.open(new InetSocketAddress("localhost", 8080));
socketChannel.configureBlocking(false);
ByteBuffer buffer = ByteBuffer.wrap("Hello, World!".getBytes());
while (buffer.hasRemaining()) {
socketChannel.write(buffer);
}
buffer.clear();
int read = socketChannel.read(buffer);
byte[] result = Arrays.copyOf(buffer.array(), read);
System.out.println(new String(result));
AIO (Asynchronous IO) 的示例代码:
AsynchronousSocketChannel socketChannel = AsynchronousSocketChannel.open();
InetSocketAddress serverAddress = new InetSocketAddress("localhost", 8080);
CompletionHandler<Void, Void> connectHandler = new CompletionHandler<>() {
@Override
public void completed(Void result, Void attachment) {
System.out.println("connected");
}
@Override
public void failed(Throwable exc, Void attachment) {
System.out.println("failed to connect");
}
};
socketChannel.connect(serverAddress, null, connectHandler);
ByteBuffer buffer = ByteBuffer.allocate(1024);
socketChannel.read(buffer, null, new CompletionHandler<>(){
@Override
public void completed(Integer result, Void attachment) {
buffer.flip();
byte[] data = new byte[buffer.limit()];
buffer.get(data);
System.out.println(new String(data));
}
@Override
public void failed(Throwable exc, Void attachment) {
System.out.println("failed to read");
}
});
阅读全文
相关推荐
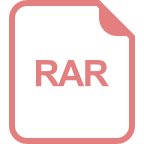
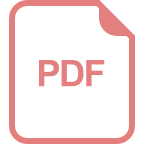


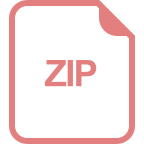
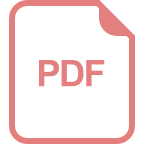
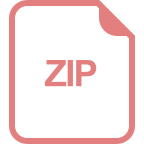
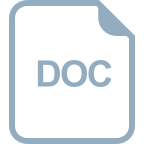


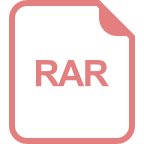
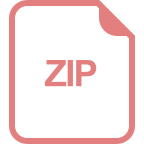
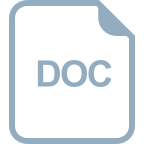




