python按照一定格式构造数据并存入文件中
时间: 2024-09-10 13:27:32 浏览: 47
在Python中,你可以使用内置的数据结构(如列表、字典、集合等)或者其他自定义的数据类来存储数据,然后将它们按照特定格式写入到文件中。这里有几个常见的文件格式示例:
1. **CSV(Comma Separated Values)**:可以使用`csv`模块来操作,比如`csv.writer`函数会生成逗号分隔的文本文件。
```python
import csv
data = [{'Name': 'Alice', 'Age': 25}, {'Name': 'Bob', 'Age': 30}]
with open('people.csv', 'w', newline='') as file:
writer = csv.DictWriter(file, fieldnames=['Name', 'Age'])
writer.writeheader()
writer.writerows(data)
```
2. **JSON**:使用`json`模块,将Python对象转换成JSON字符串,然后写入文件。
```python
import json
data = {"person": ["Alice", 25], "another_person": ["Bob", 30]}
with open('data.json', 'w') as f:
json.dump(data, f)
```
3. **XML**:可以使用`xml.etree.ElementTree`模块创建XML文档结构。
```python
import xml.etree.ElementTree as ET
root = ET.Element("data")
for person in data:
person_elem = ET.SubElement(root, "person")
name_elem = ET.SubElement(person_elem, "name")
age_elem = ET.SubElement(person_elem, "age")
name_elem.text = person['Name']
age_elem.text = str(person['Age'])
ET.ElementTree(root).write('data.xml')
```
相关推荐
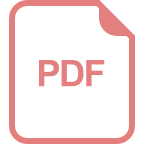
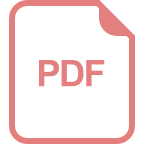
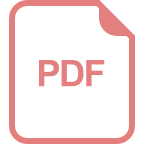














