### Problem 4: Merge Write a generator function `merge` that takes in two infinite generators `a` and `b` that are in increasing order without duplicates and returns a generator that has all the elements of both generators, in increasing order, without duplicates. > **Note:** You may assume that both `a` and `b` contains unlimited elements. As a result, calling `next(a)` and `next(b)` will never throw `StopIteration` exception. ```python def merge(a, b): """Merge two generators that are in increasing order and without duplicates. Return a generator that has all elements of both generators in increasing order and without duplicates. >>> def sequence(start, step): ... while True: ... yield start ... start += step >>> a = sequence(2, 3) # 2, 5, 8, 11, 14, ... >>> b = sequence(3, 2) # 3, 5, 7, 9, 11, 13, 15, ... >>> result = merge(a, b) # 2, 3, 5, 7, 8, 9, 11, 13, 14, 15 >>> [next(result) for _ in range(10)] [2, 3, 5, 7, 8, 9, 11, 13, 14, 15] """ "*** YOUR CODE HERE ***"
时间: 2024-02-04 18:02:43 浏览: 93
```python
def merge(a, b):
"""
Merge two generators that are in increasing order and without duplicates.
Return a generator that has all elements of both generators in increasing
order and without duplicates.
"""
a_val = next(a)
b_val = next(b)
while True:
if a_val < b_val:
yield a_val
a_val = next(a)
elif b_val < a_val:
yield b_val
b_val = next(b)
else:
yield b_val
a_val = next(a)
b_val = next(b)
```
这个函数实现了两个生成器的合并,生成器 `a` 和生成器 `b`。它首先获取两个生成器的下一个值,然后在一个无限循环中执行以下操作:
- 如果 `a` 的当前值小于 `b` 的当前值,则将 `a` 的当前值作为结果发送到调用者,并获取 `a` 的下一个值。
- 如果 `b` 的当前值小于 `a` 的当前值,则将 `b` 的当前值作为结果发送到调用者,并获取 `b` 的下一个值。
- 如果两个值相等,则只需将其中一个值作为结果发送到调用者,并获取两个生成器的下一个值。
由于两个生成器都是无限的、按递增顺序排列且没有重复项,因此我们可以安全地假设它们将永远继续生成值,而且我们不必担心将它们用尽。
阅读全文
相关推荐












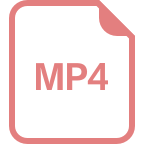
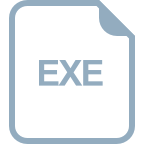




