C语言学生信息单链表代码
时间: 2024-10-23 17:00:56 浏览: 20
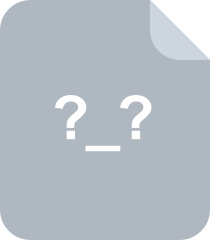
学生信息管理系统(C语言)(单链表)
在C语言中,我们可以创建一个简单的链表数据结构来存储学生的个人信息。这里是一个基本的学生信息单链表的实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义节点结构体
typedef struct {
char name[50]; // 学生姓名
int age; // 年龄
float score; // 成绩
struct Node* next; // 指向下一个节点的指针
} Student, *Node;
// 初始化一个新的节点
Node createNode(char* name, int age, float score) {
Node newNode = (Node)malloc(sizeof(Student));
if (newNode != NULL) {
strcpy(newNode->name, name);
newNode->age = age;
newNode->score = score;
newNode->next = NULL;
}
return newNode;
}
// 插入新学生到链表末尾
void insertStudent(Node* list, char* name, int age, float score) {
Node newNode = createNode(name, age, score);
if (list == NULL) {
list = newNode;
} else {
while (list->next != NULL) {
list = list->next;
}
list->next = newNode;
}
}
// 打印链表中的所有学生信息
void printList(Node* list) {
while (list != NULL) {
printf("Name: %s, Age: %d, Score: %.2f\n", list->name, list->age, list->score);
list = list->next;
}
}
int main() {
Node studentList = NULL;
// 添加一些学生
insertStudent(&studentList, "张三", 18, 90.5);
insertStudent(&studentList, "李四", 19, 85.0);
insertStudent(&studentList, "王五", 20, 92.7);
// 打印链表
printList(studentList);
return 0;
}
```
这个例子中,我们首先定义了一个`Student`结构体,包含姓名、年龄和成绩,还有一个指向下一个节点的指针。然后创建了插入节点、打印链表等功能,并在`main`函数中演示了如何操作这个链表。
阅读全文
相关推荐
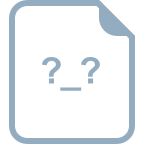
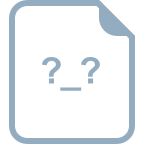
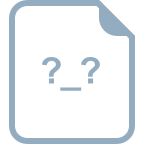
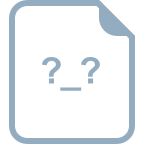
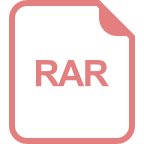
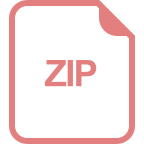
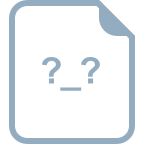
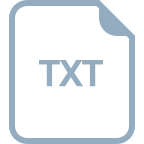
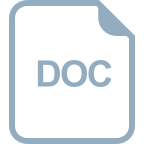
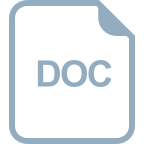
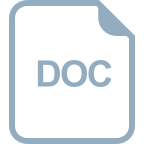


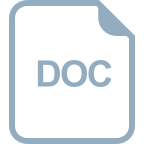
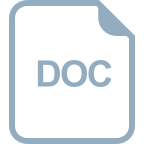
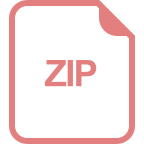