c++实现cci
时间: 2023-09-18 11:13:56 浏览: 178
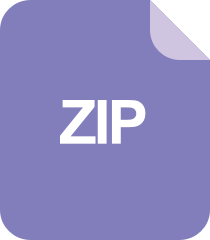
Fibonacci C++实现

以下是使用C++实现CCI指标的示例代码:
```
#include <iostream>
#include <vector>
#include <cmath>
using namespace std;
// 计算典型价格
double getTypicalPrice(double high, double low, double close) {
return (high + low + close) / 3.0;
}
// 计算平均偏差
double getMeanDeviation(vector<double> typicalPrices, double sma, int period) {
double sum = 0;
for (int i = 0; i < period; ++i) {
sum += abs(typicalPrices[i] - sma);
}
return sum / period;
}
// 计算CCI指标
double getCCI(vector<double> typicalPrices, double sma, double md, int period) {
double cci = (typicalPrices[period - 1] - sma) / (0.015 * md);
return cci;
}
int main() {
vector<double> highs = {50.12, 50.56, 50.87, 50.24, 50.17, 50.24, 50.26, 50.10, 49.87, 50.03, 49.61, 49.38, 49.55, 49.50, 49.26, 49.35, 49.92, 50.19, 50.12, 49.97, 50.03, 50.05, 49.73, 49.62, 49.50, 49.87, 50.24, 50.54, 50.50, 50.75, 50.59};
vector<double> lows = {49.50, 49.50, 50.00, 49.50, 49.50, 49.50, 49.50, 49.50, 48.62, 49.20, 48.20, 48.10, 48.68, 48.90, 48.50, 48.90, 49.20, 49.60, 49.50, 49.20, 49.50, 49.10, 49.00, 49.20, 49.00, 49.50, 49.50, 50.00, 50.20, 50.50, 50.20};
vector<double> closes = {49.87, 50.02, 50.41, 50.05, 50.09, 50.21, 50.14, 49.81, 49.68, 49.80, 48.94, 48.52, 49.02, 48.63, 48.70, 49.01, 49.75, 50.22, 50.05, 49.67, 49.93, 50.01, 49.42, 49.25, 48.98, 49.52, 50.00, 50.25, 50.52, 50.65, 50.43};
int period = 20; // CCI指标的计算周期
vector<double> typicalPrices; // 典型价格数组
double sma = 0; // 移动平均线
double md = 0; // 平均偏差
double cci = 0; // CCI指标
// 计算典型价格
for (int i = 0; i < closes.size(); ++i) {
double tp = getTypicalPrice(highs[i], lows[i], closes[i]);
typicalPrices.push_back(tp);
}
// 计算移动平均线和平均偏差
for (int i = period - 1; i < typicalPrices.size(); ++i) {
double sum = 0;
for (int j = i - period + 1; j <= i; ++j) {
sum += typicalPrices[j];
}
sma = sum / period;
md = getMeanDeviation(typicalPrices, sma, period);
// 计算CCI指标
cci = getCCI(typicalPrices, sma, md, period);
cout << "CCI(" << i + 1 << "): " << cci << endl;
}
return 0;
}
```
在上面的示例代码中,我们使用了一个长度为30的价格数组,然后计算了CCI指标。计算CCI指标时,我们首先计算了典型价格数组,然后计算移动平均线和平均偏差,最后计算CCI指标。在计算CCI指标时,我们使用了CCI指标的标准公式。
阅读全文
相关推荐
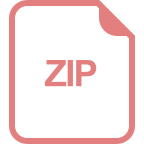
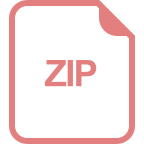
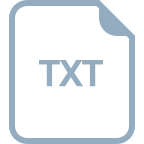
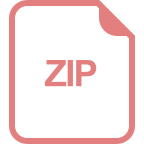
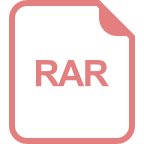
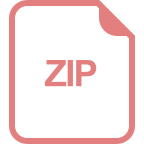
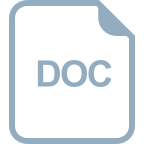
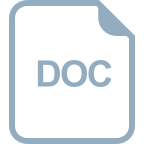
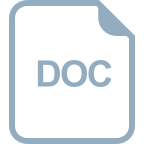


