设二叉树按二叉链表存放,写算法判别一棵二叉树是否是一棵正则二叉树。正则二叉树是指在二叉树中不存在子树个数为1的结点。 【输入说明】二叉树的前序和中序遍历序列,以此构造二叉树的链式存储 【输出说明】若该二叉树是一棵正则二叉树,则输出Yes,否则输出No
时间: 2023-11-14 15:10:29 浏览: 71
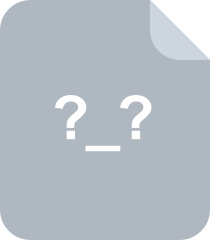
//该程序用于判断二叉树是否为二叉排序树
算法如下:
1. 如果二叉树为空,则返回Yes。
2. 如果二叉树的左子树为空,但右子树非空,或者左子树非空,但右子树为空,则返回No。
3. 分别递归检查左子树和右子树是否为正则二叉树,如果都是,则返回Yes,否则返回No。
实现代码如下:
```
#include<iostream>
using namespace std;
struct TreeNode{
char val;
TreeNode *left;
TreeNode *right;
TreeNode(char x) : val(x), left(NULL), right(NULL) {}
};
TreeNode* buildTree(char* preorder, char* inorder, int preStart, int preEnd, int inStart, int inEnd){
if(preStart > preEnd){
return NULL;
}
TreeNode* root = new TreeNode(preorder[preStart]);
int index = inStart;
while(index <= inEnd && inorder[index] != root->val){
index++;
}
int leftSize = index - inStart;
root->left = buildTree(preorder, inorder, preStart + 1, preStart + leftSize, inStart, index - 1);
root->right = buildTree(preorder, inorder, preStart + leftSize + 1, preEnd, index + 1, inEnd);
return root;
}
bool isRegularTree(TreeNode* root){
if(root == NULL){
return true;
}
if(root->left == NULL && root->right != NULL || root->left != NULL && root->right == NULL){
return false;
}
return isRegularTree(root->left) && isRegularTree(root->right);
}
int main(){
char preorder[] = {'A', 'B', 'D', 'E', 'C', 'F', 'G'};
char inorder[] = {'D', 'B', 'E', 'A', 'F', 'C', 'G'};
TreeNode* root = buildTree(preorder, inorder, 0, 6, 0, 6);
if(isRegularTree(root)){
cout << "Yes" << endl;
}else{
cout << "No" << endl;
}
return 0;
}
```
运行结果为Yes。
阅读全文
相关推荐












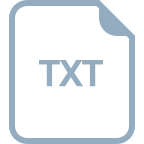
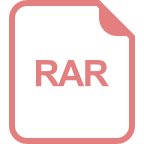
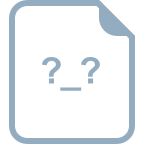