pytorch实现svm
时间: 2023-09-29 22:08:36 浏览: 70
以下是使用PyTorch实现支持向量机的基本步骤:
1. 加载数据并进行数据预处理。通常需要将数据转换为张量,并将其分成训练集和测试集。
2. 定义SVM模型。SVM模型可以通过定义一个线性模型和一个损失函数来实现。线性模型可以使用nn.Linear()函数,损失函数可以使用nn.MultiMarginLoss()函数。
3. 定义优化器。可以使用PyTorch中的优化器,例如SGD(随机梯度下降)或Adam。
4. 训练模型。在训练过程中,需要将输入数据传递给模型,并计算损失函数。然后,根据损失函数计算梯度并更新模型参数。
5. 测试模型。在测试过程中,需要将测试数据传递给模型,并计算模型的准确率。
以下是一个使用PyTorch实现SVM的示例代码:
```python
import torch
import torch.nn as nn
import torch.optim as optim
# 加载数据并进行数据预处理
x_train = torch.tensor([[0, 0], [0, 1], [1, 0], [1, 1]], dtype=torch.float32)
y_train = torch.tensor([0, 1, 1, 0], dtype=torch.float32)
# 定义SVM模型
class SVM(nn.Module):
def __init__(self):
super(SVM, self).__init__()
self.linear = nn.Linear(2, 1)
def forward(self, x):
return self.linear(x)
model = SVM()
criterion = nn.MultiMarginLoss()
optimizer = optim.SGD(model.parameters(), lr=0.1)
# 训练模型
for epoch in range(1000):
optimizer.zero_grad()
output = model(x_train)
loss = criterion(output.view(-1), y_train)
loss.backward()
optimizer.step()
# 测试模型
x_test = torch.tensor([[0, 0], [0, 1], [1, 0], [1, 1]], dtype=torch.float32)
y_test = torch.tensor([0, 1, 1, 0], dtype=torch.float32)
with torch.no_grad():
output = model(x_test)
predicted = (output > 0).int()
accuracy = (predicted == y_test).float().mean()
print("Accuracy:", accuracy.item())
```
在上述代码中,我们使用PyTorch实现了一个简单的SVM模型。该模型的输入是一个二维张量(x_train和x_test),输出是一个标量(y_train和y_test)。在训练过程中,我们使用MultiMarginLoss作为损失函数,并使用随机梯度下降(SGD)算法进行优化。最后,我们计算了模型在测试集上的准确率。
相关推荐
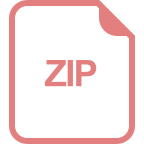
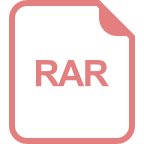
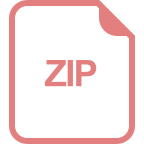














