SVM pytorch
时间: 2023-10-16 18:12:54 浏览: 41
SVM (Support Vector Machine) is a popular machine learning algorithm used for classification and regression analysis. In PyTorch, the SVM algorithm can be implemented using the torch.nn.Linear module.
The basic steps to implement SVM in PyTorch are as follows:
1. Load the data: Load the training and testing data using PyTorch's DataLoader module.
2. Define the model: Define the SVM model using the torch.nn.Linear module. The model should have two input features and one output.
3. Define the loss function: Use PyTorch's built-in loss function, torch.nn.MarginLoss, to define the loss function for the SVM model.
4. Define the optimizer: Use PyTorch's built-in optimizer, torch.optim.SGD, to define the optimizer for the SVM model.
5. Train the model: Train the SVM model using the training data and the defined loss function and optimizer.
6. Evaluate the model: Evaluate the performance of the trained SVM model using the testing data.
Here is an example code snippet for implementing SVM in PyTorch:
``` python
import torch
import torch.nn as nn
import torch.optim as optim
from torch.utils.data import DataLoader
# Load the data
train_data = DataLoader(...)
test_data = DataLoader(...)
# Define the model
class SVM(nn.Module):
def __init__(self):
super(SVM, self).__init__()
self.linear = nn.Linear(2, 1)
def forward(self, x):
return self.linear(x)
model = SVM()
# Define the loss function
criterion = nn.MarginLoss()
# Define the optimizer
optimizer = optim.SGD(model.parameters(), lr=0.01)
# Train the model
for epoch in range(num_epochs):
for inputs, labels in train_data:
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs.squeeze(), labels)
loss.backward()
optimizer.step()
# Evaluate the model
with torch.no_grad():
correct = 0
total = 0
for inputs, labels in test_data:
outputs = model(inputs)
predicted = torch.sign(outputs).squeeze()
total += labels.size(0)
correct += (predicted == labels).sum().item()
accuracy = 100 * correct / total
print(f"Accuracy: {accuracy}%")
```
In this code snippet, we define the SVM model as a subclass of nn.Module and implement the forward method to compute the output of the linear layer. We then define the loss function as nn.MarginLoss and the optimizer as optim.SGD. We train the model using a loop over the training data and compute the loss and gradients using backward and step methods. Finally, we evaluate the model using the testing data and compute the accuracy.
相关推荐
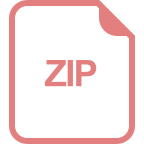
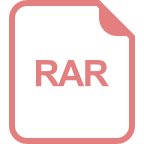
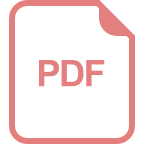














