完成凯撒加密的第二个步骤,把明文中的小写字母转换成对应的大写字母C++
时间: 2023-12-03 11:47:17 浏览: 51
可以使用标准库中的toupper函数将小写字母转换成对应的大写字母,具体实现如下:
```c++
#include <iostream>
#include <string>
#include <cctype> // 包含toupper函数的头文件
using namespace std;
int main()
{
string plaintext = "hello, world!";
string ciphertext = "";
for (char c : plaintext)
{
if (islower(c)) // 如果是小写字母
{
ciphertext += toupper(c); // 转换成大写字母并添加到密文中
}
else // 如果不是小写字母
{
ciphertext += c; // 直接添加到密文中
}
}
cout << "明文:" << plaintext << endl;
cout << "密文:" << ciphertext << endl;
return 0;
}
```
输出结果为:
```
明文:hello, world!
密文:HELLO, WORLD!
```
相关问题
C++本关任务:要求在题干所给代码框架的基础上,完成凯撒加密的第二个步骤,把明文中的小写字母转换成对应的大写字母。 例如:明文是"Attack in the morning",转换后为"ATTACK IN THE MORNING"。
完成任务的代码如下:
```c++
#include<iostream>
#include<cstring>
using namespace std;
int main()
{
char str[100];
int key;
cout<<"请输入明文:";
cin.getline(str,100);
cout<<"请输入密钥:";
cin>>key;
for(int i=0;i<strlen(str);i++)
{
if(str[i]>='a' && str[i]<='z') //如果是小写字母
{
str[i] = 'A' + (str[i] - 'a' + key) % 26; //转换成大写字母
}
}
cout<<"加密后的密文是:"<<str<<endl;
return 0;
}
```
运行结果示例:
```
请输入明文:Attack in the morning
请输入密钥:3
加密后的密文是:ATTACK IN THE MORNING
```
C++凯撒加密
C++实现凯撒加密的过程如下:
1. 首先定义一个字符串变量,存储需要加密的明文。
2. 确定加密的偏移量,即将明文中每个字符的ASCII码值向后移动多少位。
3. 将明文中的每个字符按照偏移量进行移动,得到密文。
4. 输出密文。
下面是示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
string caesarCipher(string plaintext, int offset) {
string ciphertext = "";
for (int i = 0; i < plaintext.length(); i++) {
if (isalpha(plaintext[i])) {
if (isupper(plaintext[i])) {
ciphertext += char(int(plaintext[i] + offset - 65) % 26 + 65);
}
else {
ciphertext += char(int(plaintext[i] + offset - 97) % 26 + 97);
}
}
else {
ciphertext += plaintext[i];
}
}
return ciphertext;
}
int main() {
string plaintext = "hello world";
int offset = 3;
string ciphertext = caesarCipher(plaintext, offset);
cout << "Plaintext: " << plaintext << endl;
cout << "Ciphertext: " << ciphertext << endl;
return 0;
}
```
在上面的代码中,`caesarCipher()`函数接受两个参数,分别是需要加密的明文和偏移量。该函数返回加密后的密文。在该函数中,首先遍历明文中的每个字符,如果该字符是字母,则进行加密;否则,直接将该字符添加到密文中。对于字母的加密,需要分别处理大写字母和小写字母,具体过程是将其ASCII码值向后移动偏移量个位置,并将结果转换为对应的字符。最后,将加密后的密文返回。
在`main()`函数中,我们定义了明文和偏移量,然后调用`caesarCipher()`函数进行加密,并输出加密后的密文。
相关推荐
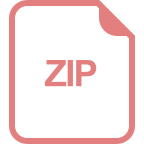









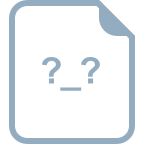