凯撒密码c++编写小白
时间: 2024-09-25 10:10:07 浏览: 12
凯撒密码是一种简单的替换加密技术,它的基本思想是将明文中的每个字符按照字母表顺序向后移动固定数量的位置作为密文。在C++中为初学者实现凯撒密码,你可以按照以下步骤操作:
1. 定义一个函数`caesar_encrypt`,接受两个参数:明文字符串`plaintext`和偏移量`shift`。这个函数将遍历输入的每个字符,如果是大写字母,就使用ASCII码值进行加密;如果是小写字母,同样处理,然后加上偏移量。
```cpp
#include <string>
std::string caesar_encrypt(const std::string& plaintext, int shift) {
std::string ciphertext = "";
for (char c : plaintext) {
if ('A' <= c && c <= 'Z') { // 大写字母
ciphertext += static_cast<char>((c - 'A' + shift) % 26 + 'A');
} else if ('a' <= c && c <= 'z') { // 小写字母
ciphertext += static_cast<char>((c - 'a' + shift) % 26 + 'a');
} else {
ciphertext += c; // 非字母字符直接保留
}
}
return ciphertext;
}
```
2. 提供一个主函数来获取用户输入并进行加密:
```cpp
int main() {
std::string input;
int shift;
std::cout << "请输入明文: ";
getline(std::cin, input);
std::cout << "请输入偏移量: ";
std::cin >> shift;
std::string encrypted_text = caesar_encrypt(input, shift);
std::cout << "加密后的文本: " << encrypted_text << std::endl;
return 0;
}
```
相关推荐
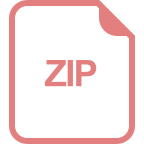
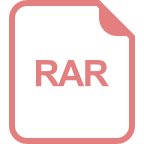
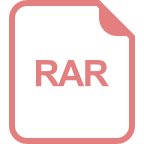
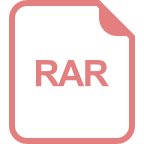
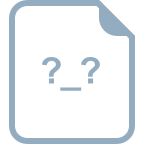
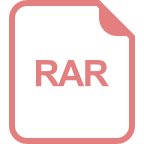
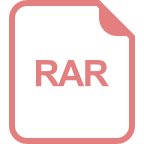
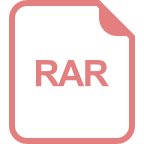






