用c++编写凯撒密码
时间: 2024-12-26 09:30:17 浏览: 13
凯撒密码是一种简单的替换式加密技术,它通过将明文中的每个字母按照固定位移进行替换来达到加密的目的。以下是用C++编写凯撒密码的一种简单示例:
```cpp
#include <iostream>
using namespace std;
// 函数:加密函数
string caesarCipherEncrypt(string plaintext, int shift) {
string ciphertext = "";
for (char c : plaintext) {
if (isalpha(c)) { // 检查字符是否为字母
// A-Z -> Z-A+shift; a-z -> z-a+shift
char encryptedChar = static_cast<char>((c + shift - 'A') % 26 + 'A');
ciphertext += encryptedChar;
} else {
ciphertext += c; // 非字母字符直接保留
}
}
return ciphertext;
}
// 函数:解密函数
string caesarCipherDecrypt(string ciphertext, int shift) {
return caesarCipherEncrypt(ciphertext, -shift);
}
int main() {
string message = "Hello, World!";
int key = 3; // 示例偏移量
// 加密
string encrypted = caesarCipherEncrypt(message, key);
cout << "Encrypted message: " << encrypted << endl;
// 解密
string decrypted = caesarCipherDecrypt(encrypted, key);
cout << "Decrypted message: " << decrypted << endl;
return 0;
}
```
在这个例子中,`caesarCipherEncrypt`函数用于加密,接收明文和偏移量作为输入;`caesarCipherDecrypt`函数则用于解密,它是对加密函数的逆操作。`main`函数中展示了如何应用这两个函数。
阅读全文
相关推荐






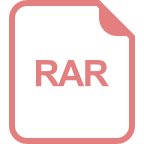










