编写c++程序实现凯撒密码的加密
时间: 2024-11-27 17:22:32 浏览: 66
凯撒密码是一种简单的替换式加密技术,通过将明文中的每个字母按照固定的偏移量向后移动来实现加密。下面是一个简单的C++程序实现凯撒密码的加密:
```cpp
#include <iostream>
#include <string>
std::string caesar_cipher_encrypt(const std::string& plaintext, int shift) {
std::string ciphertext;
for (char c : plaintext) {
if ('A' <= c && c <= 'Z') { // 处理大写字母
ciphertext += static_cast<char>((c + shift - 'A') % 26 + 'A');
} else if ('a' <= c && c <= 'z') { // 处理小写字母
ciphertext += static_cast<char>((c + shift - 'a') % 26 + 'a');
} else { // 非字母字符不变
ciphertext += c;
}
}
return ciphertext;
}
int main() {
std::string input_text = "Hello, World!";
int shift_amount = 3; // 加密偏移量
std::string encrypted_text = caesar_cipher_encrypt(input_text, shift_amount);
std::cout << "Original text: " << input_text << "\n";
std::cout << "Encrypted text: " << encrypted_text << "\n";
return 0;
}
```
在这个例子中,`caesar_cipher_encrypt`函数接收一个字符串和一个整数偏移量,对输入的每个字符进行相应的位移并返回加密后的结果。注意,这个实现只适用于英文文本,对于非字母字符不做处理。
阅读全文
相关推荐





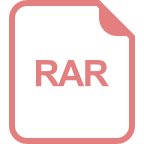
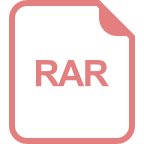












