用C++编写一个贪吃蛇小游戏的代码,并对代码进行解释
时间: 2024-01-28 10:03:27 浏览: 73
好的,下面是一个简单的C++贪吃蛇游戏的代码,包括注释解释每个部分的代码功能:
```c++
#include <iostream>
#include <conio.h> //使用getch()函数
#include <windows.h> //使用Sleep()函数
using namespace std;
bool gameOver; //游戏是否结束的标志
const int width = 20; //游戏区域的宽度
const int height = 20; //游戏区域的高度
int x, y, fruitX, fruitY, score; //蛇头、水果和分数的坐标
int tailX[100], tailY[100]; //蛇身体的坐标
int nTail; //蛇身体的长度
enum eDirection {STOP = 0, LEFT, RIGHT, UP, DOWN}; //定义方向枚举类型
eDirection dir; //蛇移动的方向
void Setup() //游戏初始化
{
gameOver = false; //游戏未结束
dir = STOP; //蛇未移动
x = width / 2; //蛇头坐标位于游戏区域中心
y = height / 2;
fruitX = rand() % width; //随机生成水果坐标
fruitY = rand() % height;
score = 0; //分数初始化为0
}
void Draw() //绘制游戏区域
{
system("cls"); //清屏
for (int i = 0; i < width+2; i++) //绘制上边界
cout << "#";
cout << endl;
for (int i = 0; i < height; i++) //绘制游戏区域
{
for (int j = 0; j < width; j++)
{
if (j == 0) //绘制左边界
cout << "#";
if (i == y && j == x) //绘制蛇头
cout << "O";
else if (i == fruitY && j == fruitX) //绘制水果
cout << "F";
else //绘制蛇身体
{
bool print = false;
for (int k = 0; k < nTail; k++)
{
if (tailX[k] == j && tailY[k] == i)
{
cout << "o";
print = true;
}
}
if (!print)
cout << " ";
}
if (j == width - 1) //绘制右边界
cout << "#";
}
cout << endl;
}
for (int i = 0; i < width+2; i++) //绘制下边界
cout << "#";
cout << endl;
cout << "Score: " << score << endl; //显示分数
}
void Input() //获取用户输入
{
if (_kbhit()) //检测键盘输入
{
switch (_getch()) //获取键盘输入
{
case 'a': //左移
dir = LEFT;
break;
case 'd': //右移
dir = RIGHT;
break;
case 'w': //上移
dir = UP;
break;
case 's': //下移
dir = DOWN;
break;
case 'x': //退出游戏
gameOver = true;
break;
}
}
}
void Logic() //游戏逻辑
{
int prevX = tailX[0]; //记录蛇尾的前一个位置
int prevY = tailY[0];
int prev2X, prev2Y;
tailX[0] = x; //蛇尾移动
tailY[0] = y;
for (int i = 1; i < nTail; i++)
{
prev2X = tailX[i];
prev2Y = tailY[i];
tailX[i] = prevX;
tailY[i] = prevY;
prevX = prev2X;
prevY = prev2Y;
}
switch (dir) //根据方向移动蛇头
{
case LEFT:
x--;
break;
case RIGHT:
x++;
break;
case UP:
y--;
break;
case DOWN:
y++;
break;
}
//判断游戏是否结束
if (x > width || x < 0 || y > height || y < 0)
gameOver = true;
for (int i = 0; i < nTail; i++)
{
if (tailX[i] == x && tailY[i] == y)
gameOver = true;
}
//判断是否吃到水果
if (x == fruitX && y == fruitY)
{
score += 10;
fruitX = rand() % width;
fruitY = rand() % height;
nTail++;
}
}
int main()
{
Setup(); //游戏初始化
while (!gameOver) //游戏循环
{
Draw(); //绘制游戏区域
Input(); //获取用户输入
Logic(); //游戏逻辑
Sleep(50); //延时50毫秒
}
return 0;
}
```
这个代码使用了简单的控制台图形界面,每个方格用一个字符表示,游戏区域由边界和游戏区域两部分组成。游戏开始时,蛇头位于游戏区域中心,随机生成一个水果坐标。当用户输入方向键时,蛇头按照指定方向移动,并且蛇身体也跟着移动。如果蛇头撞墙或者撞到了蛇身体,游戏结束。如果蛇头吃到了水果,分数加10分,并且蛇身体长度加1。
这个代码使用了一些C++的基本语法,比如变量定义、循环语句、条件语句、数组等等。同时,它也使用了一些C++标准库中的函数,比如`rand()`函数、`system()`函数、`_kbhit()`函数等等。如果您想要进一步学习C++语言和游戏编程,可以参考更多相关教程和资料。
阅读全文
相关推荐






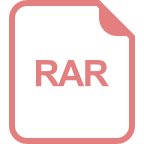
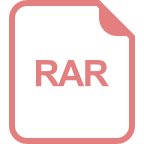









