std::map<std::string,ProfileData>g_mapProfileData;的用法
时间: 2024-09-11 13:02:20 浏览: 40
`std::map` 是 C++ 标准模板库(STL)中定义的一个关联容器,它可以存储键值对(key-value pairs),每个键都是唯一的,并且按照键的顺序(通常是二叉树的方式)来存储这些键值对。`std::map` 通常是通过红黑树来实现的,这种实现保证了插入、查找和删除操作的平均时间复杂度都是 O(log n)。
在你的代码 `std::map<std::string, ProfileData> g_mapProfileData;` 中,定义了一个名为 `g_mapProfileData` 的 `std::map` 对象,其键类型为 `std::string`(字符串),值类型为 `ProfileData`(假设这是一个结构体或类类型,这里没有给出 `ProfileData` 的定义)。
以下是一些基本的用法示例:
1. 插入数据:
```cpp
// 创建一个ProfileData类型的对象
ProfileData profile1;
// ... 给profile1赋值 ...
// 将profile1插入到map中,键为"key1"
g_mapProfileData["key1"] = profile1;
// 使用insert方法插入数据
g_mapProfileData.insert(std::make_pair("key2", profile1));
```
2. 查找数据:
```cpp
// 查找键为"key1"的数据
if (g_mapProfileData.find("key1") != g_mapProfileData.end()) {
// 如果找到了,使用迭代器访问数据
ProfileData data = g_mapProfileData["key1"];
}
```
3. 遍历map:
```cpp
// 使用迭代器遍历map中的所有元素
for(auto it = g_mapProfileData.begin(); it != g_mapProfileData.end(); ++it) {
// it->first 是键,it->second 是对应的值
std::string key = it->first;
ProfileData profile = it->second;
// ... 进行操作 ...
}
```
4. 删除数据:
```cpp
// 删除键为"key1"的数据
g_mapProfileData.erase("key1");
```
阅读全文
相关推荐
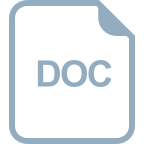
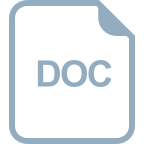
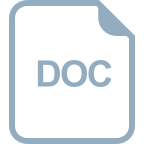

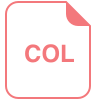
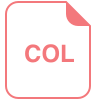
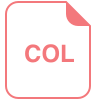
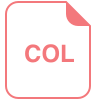
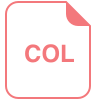
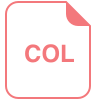
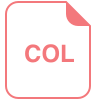
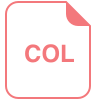
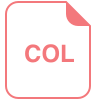
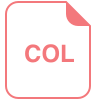
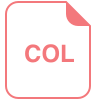
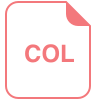
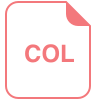
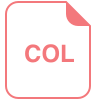
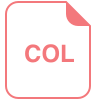