用java设计并实现一个 NextDate()函数,函数功能为:NextDate()函数包含三个变量:month 、 day 和 year ,函数的输出为输入日期后一天的日期。
时间: 2024-03-04 20:48:13 浏览: 112
好的,我可以帮你设计实现这个函数。首先需要考虑如何处理闰年和月份的天数不同的情况。以下是我的实现思路:
1. 首先判断输入日期是否合法,即月份在1-12之间,天数在1-31之间。
2. 判断该年份是否为闰年。如果是闰年,2月份有29天,否则2月份有28天。
3. 根据月份确定该月份的天数。对于1、3、5、7、8、10、12月份,每月有31天;对于4、6、9、11月份,每月有30天。
4. 判断输入日期是否为该月份的最后一天,是则将月份加1,日设为1;否则将日加1。
5. 如果月份为12,且日为31,则将年份加1,月份和日设为1。
下面是Java代码实现:
```java
public static String nextDate(int month, int day, int year) {
// 判断月份和天数是否合法
if (month < 1 || month > 12 || day < 1 || day > 31) {
return "Invalid date";
}
// 判断是否为闰年
boolean isLeapYear = false;
if (year % 4 == 0 && year % 100 != 0 || year % 400 == 0) {
isLeapYear = true;
}
// 根据月份确定天数
int daysInMonth = 0;
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
daysInMonth = 31;
break;
case 4:
case 6:
case 9:
case 11:
daysInMonth = 30;
break;
case 2:
daysInMonth = isLeapYear ? 29 : 28;
break;
}
// 判断是否为该月份的最后一天
if (day == daysInMonth) {
if (month == 12) {
year++;
month = 1;
} else {
month++;
}
day = 1;
} else {
day++;
}
// 返回结果
return String.format("%02d/%02d/%04d", month, day, year);
}
```
使用示例:
```java
String nextDate = nextDate(2, 28, 2020);
System.out.println(nextDate); // 输出:02/29/2020
nextDate = nextDate(2, 28, 2019);
System.out.println(nextDate); // 输出:03/01/2019
nextDate = nextDate(12, 31, 2020);
System.out.println(nextDate); // 输出:01/01/2021
```
希望这个示例代码能够帮助到你!
阅读全文
相关推荐
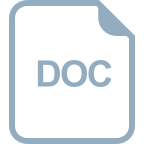
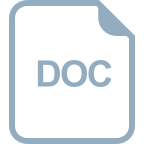
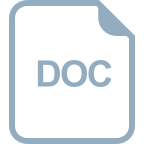














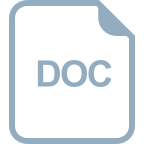
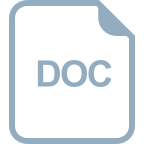
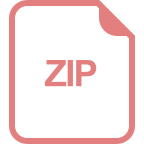