请设计一个复数类Complex,实现以下算法:int main() { Complex a, b, c; cin >> a >> b; c = a + b + 5; cout << c << endl; c = a - b - 5; cout << c << endl; c = a * b * 5; cout << c << endl; c = a / b / 5; cout << c << endl; return 0; }输出分四行,每行是一个复数,用 实部+虚部i 的方式输出,如果虚部是负数,则不要输加号,如果虚部为0,则只输出实部,如果实部为0,则只输出虚部,如果虚部绝对值为1。则只输出i 例如 1+2i 1 1-i 5i
时间: 2024-03-18 11:38:39 浏览: 19
以下是一个实现上述功能的复数类 Complex 的代码:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Complex {
public:
Complex(double r = 0.0, double i = 0.0) : real(r), imag(i) {}
friend Complex operator+(const Complex& a, const Complex& b);
friend Complex operator-(const Complex& a, const Complex& b);
friend Complex operator*(const Complex& a, const Complex& b);
friend Complex operator/(const Complex& a, const Complex& b);
friend istream& operator>>(istream& in, Complex& c);
friend ostream& operator<<(ostream& out, const Complex& c);
private:
double real, imag;
};
Complex operator+(const Complex& a, const Complex& b) {
return Complex(a.real + b.real, a.imag + b.imag);
}
Complex operator-(const Complex& a, const Complex& b) {
return Complex(a.real - b.real, a.imag - b.imag);
}
Complex operator*(const Complex& a, const Complex& b) {
return Complex(a.real * b.real - a.imag * b.imag, a.real * b.imag + a.imag * b.real);
}
Complex operator/(const Complex& a, const Complex& b) {
double divisor = b.real * b.real + b.imag * b.imag;
return Complex((a.real * b.real + a.imag * b.imag) / divisor, (-a.real * b.imag + a.imag * b.real) / divisor);
}
istream& operator>>(istream& in, Complex& c) {
in >> c.real >> c.imag;
return in;
}
ostream& operator<<(ostream& out, const Complex& c) {
if (c.real == 0 && c.imag == 0) {
out << 0;
} else if (c.real == 0) {
if (c.imag == 1) {
out << "i";
} else if (c.imag == -1) {
out << "-i";
} else {
out << c.imag << "i";
}
} else if (c.imag == 0) {
out << c.real;
} else {
if (c.imag == 1) {
out << c.real << "+i";
} else if (c.imag == -1) {
out << c.real << "-i";
} else if (c.imag > 0) {
out << c.real << "+" << c.imag << "i";
} else {
out << c.real << c.imag << "i";
}
}
return out;
}
int main() {
Complex a, b, c;
cin >> a >> b;
c = a + b + 5;
cout << c << endl;
c = a - b - 5;
cout << c << endl;
c = a * b * 5;
cout << c << endl;
c = a / b / 5;
cout << c << endl;
return 0;
}
```
在上述代码中,定义了 Complex 类,包含实部和虚部两个私有成员变量,以及加、减、乘、除等四个公有成员函数,用于实现复数的加减乘除运算;同时还定义了输入输出流的重载操作,用于从标准输入流中读取复数,以及将复数以实部+虚部i 的方式输出。在 main 函数中,定义了三个 Complex 类型的变量 a、b、c,通过 cin 从标准输入流中读入两个复数 a 和 b,然后进行加减乘除运算,并将结果赋值给 c,最后通过 cout 输出结果。
相关推荐
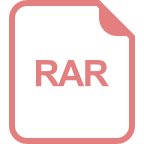
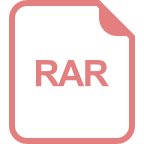
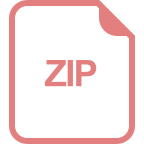









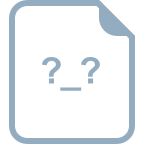
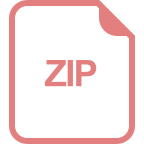
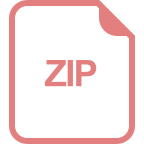
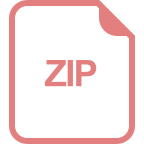
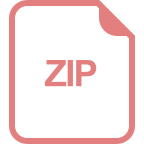