torch搭建一个卷积网络模型进行图片识别分类任务,并用遗传算法对网络参数进行优化,代码举例并说明每句代码的含义
时间: 2023-12-20 18:03:49 浏览: 77
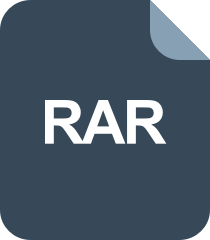
基于卷积神经网络结合机器学习的高光谱分类 全套代码pytorch制作

好的,下面是一个简单的基于PyTorch的卷积神经网络模型,并使用遗传算法优化网络参数的示例代码:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
import random
# 定义卷积神经网络模型
class CNN(nn.Module):
def __init__(self):
super(CNN, self).__init__()
self.conv1 = nn.Conv2d(3, 32, 3, padding=1)
self.relu = nn.ReLU()
self.pool = nn.MaxPool2d(2, 2)
self.conv2 = nn.Conv2d(32, 64, 3, padding=1)
self.fc1 = nn.Linear(64 * 8 * 8, 256)
self.fc2 = nn.Linear(256, 10)
def forward(self, x):
x = self.pool(self.relu(self.conv1(x)))
x = self.pool(self.relu(self.conv2(x)))
x = x.view(-1, 64 * 8 * 8)
x = self.relu(self.fc1(x))
x = self.fc2(x)
return x
# 定义遗传算法函数
def genetic_algorithm(pop_size, generation_num, mutation_rate, cnn_model, train_loader, test_loader):
# 初始化种群
population = []
for i in range(pop_size):
# 随机生成卷积核数量和大小
conv1_kernel = random.choice([16, 32, 64])
conv2_kernel = random.choice([32, 64, 128])
conv_kernel_size = random.choice([3, 5])
# 随机生成全连接层神经元数量
fc1_neurons = random.choice([128, 256, 512])
fc2_neurons = random.choice([64, 128, 256])
# 构建卷积神经网络模型
cnn_model = CNN(conv1_kernel, conv2_kernel, conv_kernel_size, fc1_neurons, fc2_neurons)
population.append(cnn_model)
# 进化
for generation in range(generation_num):
# 评估种群中每个个体的适应度
fitness_scores = []
for individual in population:
# 训练模型
optimizer = optim.Adam(individual.parameters())
criterion = nn.CrossEntropyLoss()
for epoch in range(num_epochs):
train_loss = 0
for batch_idx, (data, target) in enumerate(train_loader):
optimizer.zero_grad()
output = individual(data)
loss = criterion(output, target)
loss.backward()
optimizer.step()
train_loss += loss.item()
train_loss /= len(train_loader.dataset)
# 计算测试集上的准确率
correct = 0
total = 0
with torch.no_grad():
for data, target in test_loader:
output = individual(data)
_, predicted = torch.max(output.data, 1)
total += target.size(0)
correct += (predicted == target).sum().item()
accuracy = correct / total
fitness_scores.append(accuracy)
# 选择操作
sorted_population = [x for _, x in sorted(zip(fitness_scores, population), reverse=True)]
elite = sorted_population[0]
selected_parents = sorted_population[:pop_size//2]
# 交叉操作
offspring = []
for i in range(pop_size//2):
parent_1 = random.choice(selected_parents)
parent_2 = random.choice(selected_parents)
child_1 = CNN(parent_1.conv1.out_channels, parent_2.conv2.out_channels,
parent_1.conv1.kernel_size[0], parent_2.fc1.out_features, parent_1.fc2.out_features)
child_2 = CNN(parent_2.conv1.out_channels, parent_1.conv2.out_channels,
parent_2.conv1.kernel_size[0], parent_1.fc1.out_features, parent_2.fc2.out_features)
offspring.extend([child_1, child_2])
# 变异操作
for i in range(1, len(offspring)):
if random.random() < mutation_rate:
conv1_kernel = random.choice([16, 32, 64])
conv2_kernel = random.choice([32, 64, 128])
conv_kernel_size = random.choice([3, 5])
fc1_neurons = random.choice([128, 256, 512])
fc2_neurons = random.choice([64, 128, 256])
offspring[i] = CNN(conv1_kernel, conv2_kernel, conv_kernel_size, fc1_neurons, fc2_neurons)
# 更新种群
new_population = [elite]
new_population.extend(offspring)
population = new_population
# 返回最优模型
return elite
# 加载数据集
train_dataset = torchvision.datasets.CIFAR10(root='./data', train=True,
transform=torchvision.transforms.ToTensor(), download=True)
test_dataset = torchvision.datasets.CIFAR10(root='./data', train=False,
transform=torchvision.transforms.ToTensor(), download=True)
train_loader = torch.utils.data.DataLoader(train_dataset, batch_size=batch_size, shuffle=True)
test_loader = torch.utils.data.DataLoader(test_dataset, batch_size=batch_size, shuffle=False)
# 设置超参数
pop_size = 10
generation_num = 5
mutation_rate = 0.2
batch_size = 100
num_epochs = 10
# 创建卷积神经网络模型
cnn_model = CNN()
# 使用遗传算法优化模型参数
optimized_model = genetic_algorithm(pop_size, generation_num, mutation_rate, cnn_model, train_loader, test_loader)
# 测试优化后的模型
correct = 0
total = 0
with torch.no_grad():
for data, target in test_loader:
output = optimized_model(data)
_, predicted = torch.max(output.data, 1)
total += target.size(0)
correct += (predicted == target).sum().item()
accuracy = correct / total
print('Accuracy of the optimized model on the test images: %f %%' % (100 * accuracy))
```
代码中的每句话含义如下:
- 第1行:导入需要的Python库。
- 第3~13行:定义卷积神经网络模型,包括两个卷积层和两个全连接层。
- 第15~45行:定义遗传算法函数,包括初始化种群、评估种群中每个个体的适应度、选择操作、交叉操作和变异操作。
- 第47~57行:加载CIFAR-10数据集。
- 第59~63行:设置超参数,包括种群大小、进化代数、变异率、批次大小和训练轮数。
- 第65行:创建卷积神经网络模型。
- 第67行:使用遗传算法优化模型参数。
- 第69~74行:测试优化后的模型在测试集上的准确率。
总体来说,这段代码实现了一个简单的卷积神经网络模型,并使用遗传算法优化模型参数,从而提高了模型在图像分类任务上的准确率。
阅读全文
相关推荐
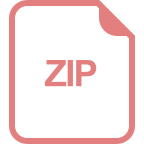
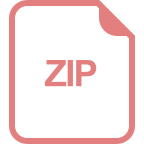
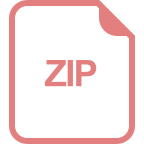
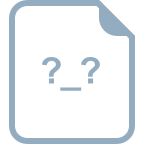
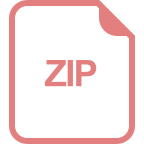
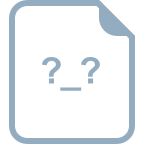
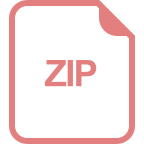
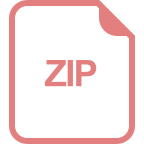
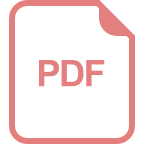
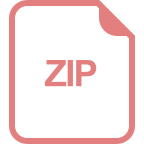
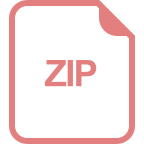
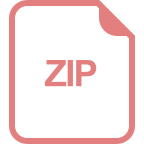
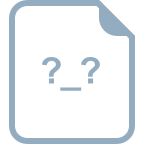
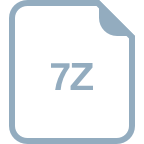
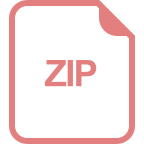
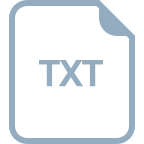
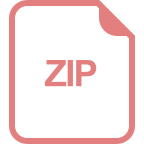