openssl + pkcs11代码
时间: 2023-08-06 13:03:30 浏览: 189
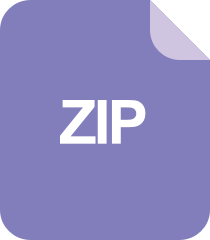
openssl_engine_pkcs11_src

以下是使用 OpenSSL 和 PKCS#11 进行加解密的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/evp.h>
#include <openssl/err.h>
#include <openssl/pkcs12.h>
#include <openssl/engine.h>
#include <openssl/pkcs11.h>
#define BUFSIZE 1024
int main(int argc, char *argv[]) {
int rv, i;
char *infile, *outfile, *password;
FILE *in, *out;
EVP_CIPHER_CTX *ctx = NULL;
PKCS12 *p12 = NULL;
X509 *cert = NULL;
EVP_PKEY *pkey = NULL;
PKCS11_CTX *p11ctx = NULL;
CK_OBJECT_HANDLE hkey;
CK_SESSION_HANDLE hsession;
if (argc != 4) {
printf("Usage: %s <infile> <outfile> <password>\n", argv[0]);
return 1;
}
infile = argv[1];
outfile = argv[2];
password = argv[3];
/* Initialize OpenSSL and PKCS#11 */
ERR_load_crypto_strings();
ENGINE_load_builtin_engines();
ENGINE_register_all_complete();
rv = PKCS11_CTX_init(&p11ctx, "pkcs11", NULL, NULL, 0);
if (rv != CKR_OK) {
printf("Error initializing PKCS#11 context\n");
goto cleanup;
}
/* Load PKCS#12 file */
in = fopen(infile, "rb");
if (in == NULL) {
printf("Error opening input file: %s\n", infile);
goto cleanup;
}
p12 = d2i_PKCS12_fp(in, NULL);
if (p12 == NULL) {
printf("Error reading PKCS#12 file: %s\n", infile);
goto cleanup;
}
fclose(in);
/* Extract certificate and private key from PKCS#12 file */
rv = PKCS12_parse(p12, password, &pkey, &cert, NULL);
if (rv == 0) {
printf("Error parsing PKCS#12 file: %s\n", infile);
goto cleanup;
}
/* Find corresponding private key in PKCS#11 token */
rv = PKCS11_CTX_login(p11ctx, CKU_USER, password);
if (rv != CKR_OK) {
printf("Error logging in to PKCS#11 token\n");
goto cleanup;
}
hsession = PKCS11_CTX_get_session(p11ctx);
rv = PKCS11_find_key(p11ctx, &hkey, cert, pkey);
if (rv != CKR_OK) {
printf("Error finding private key in PKCS#11 token\n");
goto cleanup;
}
/* Initialize context for decryption */
ctx = EVP_CIPHER_CTX_new();
if (ctx == NULL) {
printf("Error creating cipher context\n");
goto cleanup;
}
rv = EVP_OpenInit(ctx, EVP_aes_256_cbc(), NULL, 0, NULL, pkey);
if (rv != 1) {
printf("Error initializing cipher context\n");
goto cleanup;
}
/* Decrypt input file and write output file */
in = fopen(infile, "rb");
if (in == NULL) {
printf("Error opening input file: %s\n", infile);
goto cleanup;
}
out = fopen(outfile, "wb");
if (out == NULL) {
printf("Error opening output file: %s\n", outfile);
goto cleanup;
}
while (1) {
unsigned char inbuf[BUFSIZE], outbuf[BUFSIZE];
int inlen, outlen;
inlen = fread(inbuf, 1, BUFSIZE, in);
if (inlen == 0) {
break;
}
rv = EVP_OpenUpdate(ctx, outbuf, &outlen, inbuf, inlen);
if (rv != 1) {
printf("Error decrypting input file\n");
goto cleanup;
}
fwrite(outbuf, 1, outlen, out);
}
rv = EVP_OpenFinal(ctx, NULL, 0);
if (rv != 1) {
printf("Error finalizing cipher context\n");
goto cleanup;
}
fclose(in);
fclose(out);
printf("Decryption successful\n");
cleanup:
if (ctx != NULL) {
EVP_CIPHER_CTX_free(ctx);
}
if (p12 != NULL) {
PKCS12_free(p12);
}
if (cert != NULL) {
X509_free(cert);
}
if (pkey != NULL) {
EVP_PKEY_free(pkey);
}
if (p11ctx != NULL) {
PKCS11_CTX_logout(p11ctx);
PKCS11_CTX_uninit(p11ctx);
}
ENGINE_cleanup();
ERR_free_strings();
return 0;
}
```
这是一个简单的解密示例,它可以从 PKCS#12 文件中提取证书和私钥,并使用 PKCS#11 模块从智能卡中获取私钥,然后使用 OpenSSL 中的 EVP 函数进行解密操作。你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
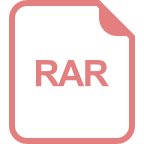
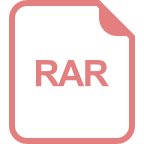
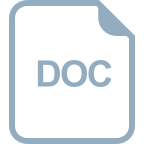





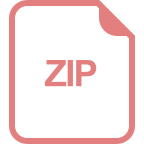
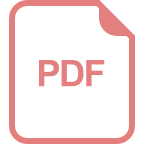
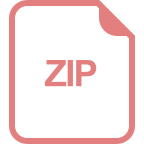



