springboot集成amazonaws实现上传下载
时间: 2024-11-16 07:27:55 浏览: 18
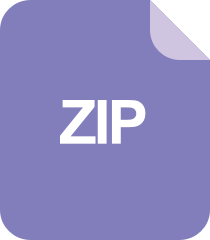
springboot集成amazon aws s3对象存储sdk(javav2)

Spring Boot 集成 Amazon AWS 实现文件上传和下载通常涉及到使用 Amazon S3(Simple Storage Service),这是 Amazon Web Services 提供的一种云端存储服务。以下是基本步骤:
1. **设置依赖**:
- 在你的`pom.xml`或`build.gradle`文件中添加Amazon SDK for Java S3的依赖,例如:
```xml
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-java-sdk-s3</artifactId>
<version>版本号</version>
</dependency>
```
或者 Gradle:
```gradle
implementation 'com.amazonaws:aws-java-sdk-s3:版本号'
```
2. **配置AWS客户端**:
- 创建一个 `application.properties` 或 `application.yml` 文件,配置AWS访问密钥、秘密访问密钥和S3桶名:
```properties
aws.accessKeyId=your_access_key
aws.secretAccessKey=your_secret_key
s3.bucketName=your_bucket_name
```
3. **创建Service类**:
- 创建一个 `AmazonS3Client` 的实例,并处理异常:
```java
@Autowired
private AmazonS3 s3Client;
public String uploadFileToS3(File file) {
try {
PutObjectRequest request = new PutObjectRequest(bucketName, file.getName(), new File(file.getAbsolutePath()));
s3Client.putObject(request);
return "Upload successful";
} catch (Exception e) {
throw new RuntimeException("Error uploading file", e);
}
}
public InputStream downloadFileFromS3(String fileName) {
try {
S3Object object = s3Client.getObject(new GetObjectRequest(bucketName, fileName));
return object.getObjectContent();
} catch (Exception e) {
throw new RuntimeException("Error downloading file", e);
}
}
```
4. **Controller接口**:
- 在控制器里,你可以提供 RESTful API 接口来上传和下载文件:
```java
@PostMapping("/upload")
public ResponseEntity<?> upload(@RequestParam("file") MultipartFile file) {
// Call the service method to upload and get the response
String response = uploadFileToS3(file.getFile());
return new ResponseEntity<>(response, HttpStatus.OK);
}
@GetMapping("/download/{fileName}")
public ResponseEntity<InputStreamResource> downloadFile(@PathVariable String fileName) {
InputStream inputStream = downloadFileFromS3(fileName);
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
return new ResponseEntity<>(new InputStreamResource(inputStream), headers, HttpStatus.OK);
}
```
阅读全文
相关推荐
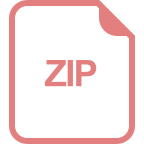
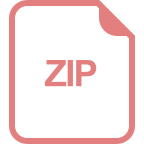

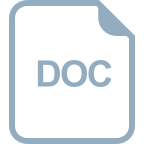
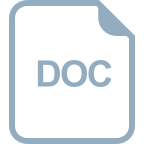
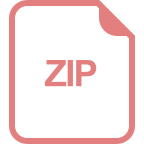
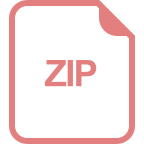
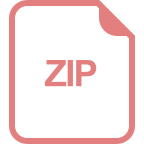
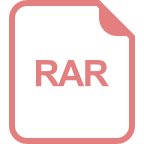
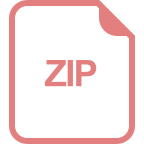
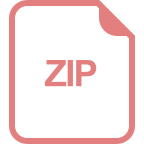
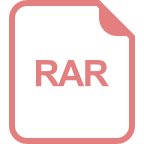
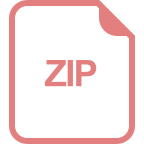
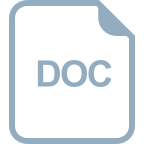




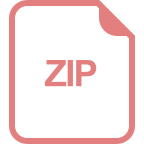