牛顿法解非线性方程组python
时间: 2023-11-03 12:01:21 浏览: 143
牛顿法是一种用于解非线性方程组的迭代方法。在Python中,可以使用scipy库的optimize模块中的root函数来实现牛顿法解非线性方程组。以下是一个示例代码:
```python
from scipy.optimize import root
# 定义一个包含多个方程的非线性方程组
def equations(x):
# 方程1: x + y - 3 = 0
eq1 = x[0] + x[1] - 3
# 方程2: x^2 + y^2 - 4 = 0
eq2 = x[0]**2 + x[1]**2 - 4
return [eq1, eq2]
# 使用牛顿法求解非线性方程组
solution = root(equations, [0, 0])
x = solution.x
# 打印解
print("x =", x[0])
print("y =", x[1])
```
相关问题
牛顿法解非线性方程组Python
牛顿法是一种用于求解非线性方程组的迭代方法。它通过线性化方程组并迭代逼近根的方法来求解非线性方程组。牛顿法的基本思想是使用当前点对应的切线来逼近曲线,然后通过求取切线与x轴的交点作为下一个迭代点,重复这个过程直至收敛到方程组的解。
在Python中,我们可以通过以下步骤使用牛顿法解非线性方程组:
1. 定义非线性方程组的函数F(x)。
2. 定义非线性方程组的Jacobi矩阵J(x)。
3. 初始化初始点x0。
4. 迭代计算下一个点x1 = x0 - J(x0)^(-1) * F(x0)。
5. 判断迭代是否收敛,如果不收敛则返回第4步,直至收敛。
6. 返回收敛的解x1。
以下是使用Python代码实现牛顿法解非线性方程组的示例:
```python
import numpy as np
def newton_method(F, J, x0, tol=1e-6, max_iter=100):
iter_count = 0
while iter_count < max_iter:
delta_x = np.linalg.solve(J(x0), -F(x0))
x1 = x0 + delta_x
if np.linalg.norm(delta_x) < tol:
return x1
x0 = x1
iter_count += 1
return None
# 示例非线性方程组的函数定义
def F(x):
return np.array([
x[0]**2 + x[1]**2 - 1,
x[0] - x[1]**2
])
# 示例非线性方程组的Jacobi矩阵定义
def J(x):
return np.array([
[2*x[0], 2*x[1]],
[1, -2*x[1]]
])
# 初始点
x0 = np.array([0.5, 0.5])
# 使用牛顿法解非线性方程组
solution = newton_method(F, J, x0)
print("解:", solution)
```
python牛顿法解非线性方程组
可以使用Python中的牛顿法来求解非线性方程组。牛顿法是一种迭代的方法,通过逐步逼近函数的根。在Python中,可以使用scipy.optimize库中的fsolve函数来实现牛顿法求解非线性方程组。
首先,需要定义一个函数,该函数返回一个数组,数组的元素是非线性方程组的各个方程的值。然后,使用fsolve函数传入定义的函数和一个初始的猜测解来求解非线性方程组。fsolve函数会返回一个数组,数组的元素是非线性方程组的解。
下面是一个使用牛顿法求解非线性方程组的示例代码:
```python
from scipy.optimize import fsolve
# 定义非线性方程组
def equations(x):
# x和x是未知数
y1 = 2*x - x - 2
y2 = x # 初始猜测解
result = fsolve(equations, x0)
print(result)
```
在上述代码中,我们定义了一个非线性方程组,包含两个方程。然后,使用fsolve函数传入定义的函数和一个初始的猜测解来求解方程组。最后,打印出求解结果。
请注意,对于复杂的非线性方程组,牛顿法的收敛性可能<span class="em">1</span>
#### 引用[.reference_title]
- *1* [基于Python利用Newton-Raphson方法求解非线性方程组](https://blog.csdn.net/weixin_39964552/article/details/84331937)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
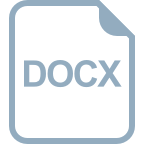
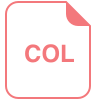
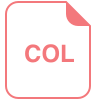
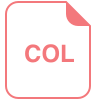
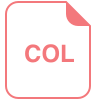
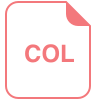







