vue上传图片和一个数组到python后端,python后端接收图片并下载到本地,前后端的代码怎么写
时间: 2024-03-05 17:49:34 浏览: 21
好的,针对您的问题,我将分别给出前端Vue和后端Python的示例代码。
前端Vue代码:
```html
<template>
<div>
<input type="file" ref="file" @change="handleFileChange" />
<button @click="upload">上传</button>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
file: null,
arr: [1, 2, 3, 4, 5],
};
},
methods: {
handleFileChange(e) {
this.file = e.target.files[0];
},
async upload() {
const formData = new FormData();
formData.append('file', this.file);
formData.append('arr', JSON.stringify(this.arr));
try {
const res = await axios.post('/api/upload', formData);
console.log(res.data);
} catch (e) {
console.error(e);
}
},
},
};
</script>
```
上面的代码中,我们使用了`<input type="file">`元素来选择文件,然后通过`FormData`来构建请求体,最后使用`axios`库发送POST请求到后端。
后端Python代码:
```python
from flask import Flask, jsonify, request
import os
app = Flask(__name__)
@app.route('/api/upload', methods=['POST'])
def upload():
file = request.files['file']
arr = request.form.get('arr')
arr = json.loads(arr)
filename = file.filename
file.save(os.path.join('/path/to/save/', filename))
return jsonify({'msg': 'success'})
if __name__ == '__main__':
app.run()
```
上面的代码中,我们使用了Flask框架来创建 Web 应用,接收前端发送的POST请求,从中获取文件和数组,然后将文件保存到本地指定路径。请注意,`/path/to/save/`需要替换为您本地存储文件的路径。
相关推荐
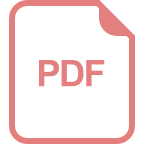
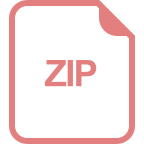
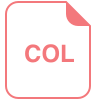










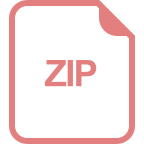
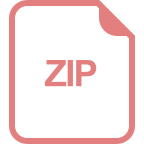
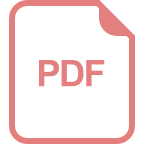
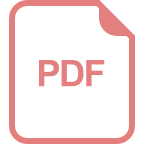