用Python生成不重复的字符
时间: 2024-04-25 20:05:56 浏览: 15
可以使用Python中的random模块和string模块来生成不重复的字符。具体实现如下:
```python
import random
import string
def generate_unique_chars(length):
# 生成长度为length的随机字符串
chars = ''.join(random.choices(string.ascii_letters + string.digits, k=length))
# 判断字符串中是否有重复字符
while len(set(chars)) != length:
chars = ''.join(random.choices(string.ascii_letters + string.digits, k=length))
return chars
```
这个函数会生成指定长度的随机字符串,并且保证字符串中没有重复的字符。你可以调用这个函数来生成不重复的字符。
相关问题
python生成36位长度的不重复字符串
可以使用Python的uuid模块来生成不重复的36位长度字符串,示例如下:
```python
import uuid
unique_str = str(uuid.uuid4())
print(unique_str)
```
输出:
```
'f0a3c5e3-eb2a-4e7d-bb40-ec330a6ed3d2'
```
其中,uuid4()方法生成一个随机的UUID,使用str()方法将其转换为字符串类型即可。
python3对字符不重复全排列
在Python3中,可以通过使用itertools库中的permutations函数来生成给定字符集合的不重复全排列。
首先,需要将要生成排列的字符集合转化为一个字符列表。例如,如果要对字符集合{'a', 'b', 'c'}进行排列,可以使用如下代码:
```
chars = ['a', 'b', 'c']
```
接着,可以使用itertools库中的permutations函数来生成排列。需要注意的是,permutations函数返回的是一个迭代器,因此需要将其转化成一个列表(或使用for循环遍历迭代器)。
```
import itertools
perms = itertools.permutations(chars)
perms_list = list(perms)
```
生成的perms_list中保存了给定字符集合的不重复全排列。在本例中,perms_list包含了下面的6个排列:
```
[('a', 'b', 'c'), ('a', 'c', 'b'), ('b', 'a', 'c'), ('b', 'c', 'a'), ('c', 'a', 'b'), ('c', 'b', 'a')]
```
需要注意的是,这些排列中不存在重复的元素。如果要生成包含重复元素的全排列,可以使用itertools库中的product函数。例如,要生成长度为3的由{'a', 'b', 'c'}中的元素组成的所有排列(可以重复)的代码如下:
```
perms_with_repeats = itertools.product(chars, repeat=3)
perms_with_repeats_list = list(perms_with_repeats)
```
生成的perms_with_repeats_list中包含了27个元素,代表了所有的排列组合。
相关推荐
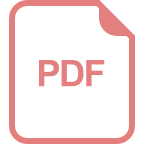
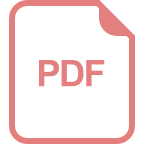











