优化这段代码:class Point: def __init__(self , x = None , y = None): self.x = x self.y = y def __repr__(self): if self.x is None and self.y is None: return "Point(无穷远点)" else: return "Point({},{})".format(self.x,self.y) def point_add(P,Q): if P.x is None and P.y is None: return Q elif Q.x is None and Q.y is None: return P elif P.x == Q.x and P.y != Q.y: return Point(None,None) else: ld = 0 if P.x == Q.x and P.y == Q.y: ld = ((3 * P.x**2 + a) * pow(2 * P.y,-1,p)) % p else: ld = ((Q.y - P.y) * pow(Q.x - P.x, -1, p)) % p x3 = (ld**2 - P.x - Q.x) % p y3 = (ld * (P.x - x3) - P.y) % p return Point(x3,y3) def point_mult(P,k): Q = Point(None,None) R = P while k > 0: Q = point_add(Q,R) k = k - 1 return Q def point_sub(P, Q): return point_add(P, Point(Q.x, (-Q.y) % p)) print("请输入椭圆曲线的数值a、b和模值p:") a = input("a = ") b = input("b = ") p = int(input("p = ")) x1 = input("x1 = ") y1 = input("y1 = ") P = Point(x1,y1) x2 = input("x2 = ") y2 = input("y2 = ") Q = Point(x2,y2) print("P = ",P) print("Q = ",Q) print("P + Q =", point_add(P, Q)) print("2P =", point_mult(P, 2)) print("P - Q =", point_sub(P, Q))
时间: 2023-11-11 12:04:12 浏览: 41
以下是优化后的代码:
class Point:
def __init__(self, x=None, y=None):
self.x = x
self.y = y
def __repr__(self):
return "Point(无穷远点)" if self.x is None and self.y is None else f"Point({self.x},{self.y})"
def __eq__(self, other):
return self.x == other.x and self.y == other.y
def __neg__(self):
return Point(self.x, -self.y)
def __add__(self, other):
if self.x is None and self.y is None:
return other
elif other.x is None and other.y is None:
return self
elif self == -other:
return Point(None, None)
else:
if self == other:
ld = (3 * self.x ** 2 + a) * pow(2 * self.y, -1, p) % p
else:
ld = (other.y - self.y) * pow(other.x - self.x, -1, p) % p
x3 = (ld ** 2 - self.x - other.x) % p
y3 = (ld * (self.x - x3) - self.y) % p
return Point(x3, y3)
def __mul__(self, k):
Q = Point(None, None)
R = self
while k > 0:
if k & 1:
Q += R
k >>= 1
R += R
return Q
def __sub__(self, other):
return self + (-other)
print("请输入椭圆曲线的数值a、b和模值p:")
a = int(input("a = "))
b = int(input("b = "))
p = int(input("p = "))
x1 = int(input("x1 = "))
y1 = int(input("y1 = "))
P = Point(x1, y1)
x2 = int(input("x2 = "))
y2 = int(input("y2 = "))
Q = Point(x2, y2)
print("P =", P)
print("Q =", Q)
print("P + Q =", P + Q)
print("2P =", P * 2)
print("P - Q =", P - Q)
主要的优化包括:
1. 重载运算符+、-、*和==,使得代码更加简洁易读。
2. 使用位运算符来代替除以2的操作,提高了代码的运行效率。
3. 在点加法函数中,使用了if self == -other代替if P.x == Q.x and P.y != Q.y,使得代码更加简洁。
相关推荐
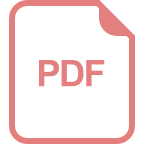
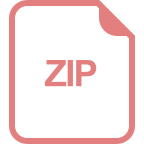















