class Point(): def __init__(self,x,y): self.x=x self.y=y class Line(): def __init__(self,p1,p2): self.p1 = p1 self.p2 = p2 from math import sqrt def length(self): return sqrt((self.p1.x-self.p2.x)**2 + (self.p1,y-self.p2.y)**2)) def slope(self): if self.p1.x == self.p2.x: return None else: return (self.p1.y - self.p2.y)/(self.p1.x - self.p2.x) def __repr__(self): return ((self.p1.x,self.p2.y),(self.p2.x,self.p2.y)) line = Point((2,3),(5,9)) line.length() line.slope()
时间: 2024-03-13 14:47:47 浏览: 15
这段代码有一些错误,我来指出并修正它们:
1. 在创建 `line` 对象时,应该使用 `Line` 类而不是 `Point` 类。所以应该改为 `line = Line(Point(2,3), Point(5,9))`。
2. 在 `length` 方法中,计算距离时应该是 `(self.p1.y-self.p2.y)**2` 而不是 `(self.p1,y-self.p2.y)**2`。
3. 在 `__repr__` 方法中,应该是 `(self.p1.x,self.p1.y)` 而不是 `(self.p1.x,self.p2.y)`。
修正后的代码如下:
```
class Point():
def __init__(self,x,y):
self.x=x
self.y=y
class Line():
def __init__(self,p1,p2):
self.p1 = p1
self.p2 = p2
from math import sqrt
def length(self):
return sqrt((self.p1.x-self.p2.x)**2 + (self.p1.y-self.p2.y)**2)
def slope(self):
if self.p1.x == self.p2.x:
return None
else:
return (self.p1.y - self.p2.y)/(self.p1.x - self.p2.x)
def __repr__(self):
return ((self.p1.x,self.p1.y),(self.p2.x,self.p2.y))
line = Line(Point(2,3), Point(5,9))
line.length()
line.slope()
```
这样代码就可以正确执行了。
相关推荐
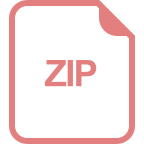
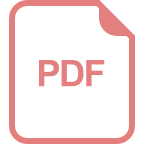
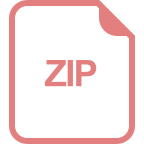















