class Point: def __init__(self, x, y): self.__x = x self.__y = y def __add__(self, other): x = self.__x + other.__x y = self.__y + other.__y return Point(x, y) def __sub__(self, other): x = self.__x - other.__x y = self.__y - other.__y return Point(x, y) def __mul__(self, a): x = self.__x * a y = self.__y * a return Point(x, y) def __truediv__(self, a): x = self.__x / a y = self.__y / a return Point(x, y) r1 = Point(10, 20) r2 = Point(5, 5) print(r1+r2) print(r1-r2) print(r1*2) print(r1/2)代码改进,输出向量坐标
时间: 2023-11-11 17:04:55 浏览: 239
为了输出向量坐标,我们可以在 Point 类中添加一个方法来返回坐标的元组,如下所示:
```python
class Point:
def __init__(self, x, y):
self.__x = x
self.__y = y
def __add__(self, other):
x = self.__x + other.__x
y = self.__y + other.__y
return Point(x, y)
def __sub__(self, other):
x = self.__x - other.__x
y = self.__y - other.__y
return Point(x, y)
def __mul__(self, a):
x = self.__x * a
y = self.__y * a
return Point(x, y)
def __truediv__(self, a):
x = self.__x / a
y = self.__y / a
return Point(x, y)
def get_coordinates(self):
return (self.__x, self.__y)
```
然后我们就可以使用这个方法来输出向量坐标了,示例如下:
```python
r1 = Point(10, 20)
r2 = Point(5, 5)
print(r1+r2.get_coordinates()) # 输出 (15, 25)
print(r1-r2.get_coordinates()) # 输出 (5, 15)
print(r1*2).get_coordinates()) # 输出 (20, 40)
print(r1/2).get_coordinates()) # 输出 (5.0, 10.0)
```
阅读全文
相关推荐
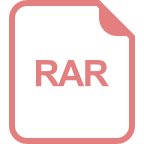
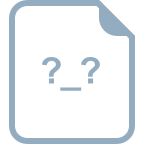
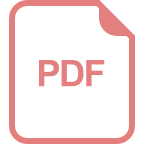
















