class SelfAttention(nn.Module): def __init__(self, in_channels, reduction=4): super(SelfAttention, self).__init__() self.avg_pool = nn.AdaptiveAvgPool1d(1) # print("in_channels", in_channels) print("in_channels:", in_channels) print("reduction:", reduction) self.fc1 = nn.Conv1d(in_channels, in_channels // reduction, 1, bias=False) self.relu = nn.ReLU(inplace=True) self.fc2 = nn.Conv1d(in_channels // reduction, in_channels, 1, bias=False) self.sigmoid = nn.Sigmoid() def forward(self, x): b, c, n = x.size() print("x.shape=", x.shape) y = self.avg_pool(x) print("y.shape=", y.shape) # print("channel_out", channel_out) y = self.fc1(y) y = self.relu(y) y = self.fc2(y) y = self.sigmoid(y) return x * y.expand_as(x) def get_model(input_channels=6, use_xyz=True): return Pointnet2MSG(input_channels=input_channels, use_xyz=use_xyz) class Pointnet2MSG(nn.Module): def __init__(self, input_channels=6, use_xyz=True): super(Pointnet2MSG, self).__init__() self.SA_modules = nn.ModuleList() channel_in = input_channels # print("channel_in=", channel_in) skip_channel_list = [input_channels] for k in range(cfg.RPN.SA_CONFIG.NPOINTS.__len__()): mlps = cfg.RPN.SA_CONFIG.MLPS[k].copy() channel_out = 0 # print("channel_in=", channel_in) for idx in range(mlps.__len__()): mlps[idx] = [channel_in] + mlps[idx] channel_out += mlps[idx][-1] mlps.append(channel_out) # print(mlps) print("channel_out", channel_out) self.SA_modules.append( nn.Sequential( PointnetSAModuleMSG( npoint=cfg.RPN.SA_CONFIG.NPOINTS[k], radii=cfg.RPN.SA_CONFIG.RADIUS[k], nsamples=cfg.RPN.SA_CONFIG.NSAMPLE[k], mlps=mlps[:2], use_xyz=use_xyz, bn=cfg.RPN.USE_BN, ), SelfAttention(channel_out) ) ) skip_channel_list.append(channel_out) channel_in = channel_out根据以上代码块,自注意力机制模块中forward函数所接受的x参数的值到底是什么?
时间: 2023-11-20 18:05:53 浏览: 148
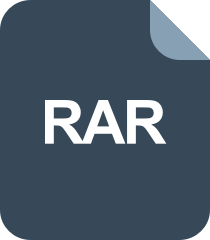
PAPR.rar_PAPR_in_ofdm papr_ofdm papr reduction_papr reduction

根据代码块中的描述,自注意力机制模块中forward函数所接受的x参数应该是输入的特征张量,其形状为(b, c, n),其中b表示batch size,c表示通道数,n表示点云中点的数目。在代码块中,x首先通过avg_pool进行自适应平均池化操作得到y,然后经过fc1、relu、fc2和sigmoid层得到注意力权重,最后将输入特征张量x与注意力权重y相乘得到加权特征张量作为输出。
阅读全文