spring boot实现通过波特率、站地址、数据位、停止位等信息和串口进行通信并发送接收数据的完整代码实现
时间: 2023-12-24 16:05:35 浏览: 121
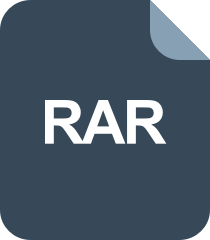
串口调试源代码,实现串口数据的发送、接收

好的,这是一个简单的Spring Boot串口通信的例子,你可以根据自己的需要进行修改:
首先,你需要在你的pom.xml文件中添加如下依赖:
```xml
<dependency>
<groupId>com.fazecast</groupId>
<artifactId>jSerialComm</artifactId>
<version>2.6.2</version>
</dependency>
```
然后,你需要创建一个SerialService类,用于实现串口通信的相关操作:
```java
import com.fazecast.jSerialComm.SerialPort;
import org.springframework.stereotype.Service;
import javax.annotation.PostConstruct;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
@Service
public class SerialService {
private SerialPort serialPort;
private OutputStream outputStream;
private InputStream inputStream;
@PostConstruct
public void init() {
// 设置串口参数
serialPort = SerialPort.getCommPort("COM1");
serialPort.setComPortParameters(9600, 8, 1, SerialPort.NO_PARITY);
// 打开串口
if (serialPort.openPort()) {
System.out.println("串口已打开");
} else {
System.out.println("串口打开失败");
return;
}
// 获取输入输出流
outputStream = serialPort.getOutputStream();
inputStream = serialPort.getInputStream();
// 启动读取线程
Thread thread = new Thread(() -> {
while (true) {
try {
// 读取数据
byte[] buffer = new byte[1024];
int len = inputStream.read(buffer);
if (len > 0) {
String data = new String(buffer, 0, len);
System.out.println("接收到数据:" + data);
}
} catch (IOException e) {
e.printStackTrace();
}
}
});
thread.setDaemon(true);
thread.start();
}
/**
* 发送数据
*
* @param data
*/
public void send(String data) {
try {
outputStream.write(data.getBytes());
outputStream.flush();
System.out.println("发送数据:" + data);
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 关闭串口
*/
public void close() {
if (serialPort != null && serialPort.isOpen()) {
serialPort.closePort();
System.out.println("串口已关闭");
}
}
}
```
在这个类中,我们通过jSerialComm库来实现串口的打开、关闭、读取和写入等操作。在初始化方法(@PostConstruct)中,我们设置了串口参数并打开了串口,通过获取输入输出流来实现数据的读取和写入。启动了一个读取线程,用于不断地从串口读取数据。
接下来,你需要创建一个Controller类,用于调用SerialService类中的方法:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/serial")
public class SerialController {
@Autowired
private SerialService serialService;
/**
* 发送数据
*
* @param data
* @return
*/
@PostMapping("/send")
public String send(@RequestParam String data) {
serialService.send(data);
return "发送成功";
}
/**
* 关闭串口
*
* @return
*/
@PostMapping("/close")
public String close() {
serialService.close();
return "关闭成功";
}
}
```
在这个Controller中,我们通过注入SerialService类来调用方法,实现数据的发送和串口的关闭。
最后,你需要在你的Spring Boot应用程序中添加@Configuration注解来启用Spring Boot的自动配置功能:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
这样,你就可以通过访问API接口来发送数据和关闭串口了。
阅读全文
相关推荐
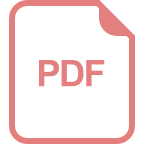
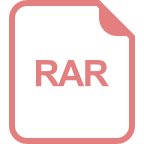
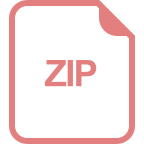
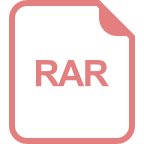
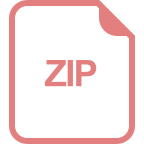
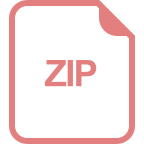
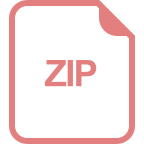
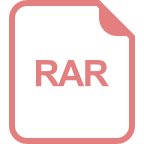
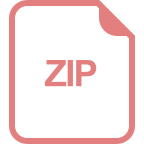
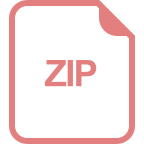
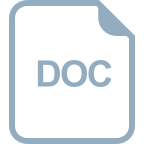
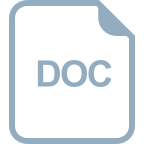
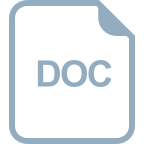



