三轴加速度 计算步数,c语言程序
时间: 2023-06-27 12:06:19 浏览: 152
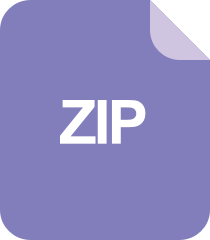
三轴加速度传感器计步测算
计算步数的算法通常有很多种,以下是一种简单的基于三轴加速度的计步算法的 C 语言程序:
```c
#include <stdio.h>
#include <math.h>
#define FILTER_ALPHA 0.3 // 低通滤波器的 alpha 值
#define PEAK_THRESHOLD 1.5 // 步数峰值的阈值
#define WINDOW_SIZE 20 // 步数峰值检测的窗口大小
int main() {
// 初始化
int step_count = 0; // 步数计数器
float x_acc, y_acc, z_acc; // 三轴加速度
float x_acc_filtered = 0, y_acc_filtered = 0, z_acc_filtered = 0; // 低通滤波后的加速度
float acc_magnitude = 0, acc_magnitude_filtered = 0; // 加速度模和滤波后的加速度模
float acc_magnitude_max = -INFINITY; // 步数峰值检测的最大加速度模
int peak_detected = 0; // 步数峰值是否被检测到的标志
int window[WINDOW_SIZE] = {0}; // 步数峰值检测的滑动窗口
// 循环读取加速度数据并计算步数
while (1) {
// 读取加速度数据(这里假设已经有相应的函数)
read_acc_data(&x_acc, &y_acc, &z_acc);
// 低通滤波
x_acc_filtered = FILTER_ALPHA * x_acc + (1 - FILTER_ALPHA) * x_acc_filtered;
y_acc_filtered = FILTER_ALPHA * y_acc + (1 - FILTER_ALPHA) * y_acc_filtered;
z_acc_filtered = FILTER_ALPHA * z_acc + (1 - FILTER_ALPHA) * z_acc_filtered;
// 计算加速度模
acc_magnitude = sqrt(x_acc * x_acc + y_acc * y_acc + z_acc * z_acc);
acc_magnitude_filtered = sqrt(x_acc_filtered * x_acc_filtered + y_acc_filtered * y_acc_filtered + z_acc_filtered * z_acc_filtered);
// 步数峰值检测
if (!peak_detected) {
// 把当前的加速度模放入滑动窗口
for (int i = WINDOW_SIZE - 1; i > 0; i--) {
window[i] = window[i - 1];
}
window[0] = acc_magnitude_filtered;
// 检查滑动窗口的最大值是否超过阈值
if (acc_magnitude_filtered > acc_magnitude_max) {
acc_magnitude_max = acc_magnitude_filtered;
}
if (window[WINDOW_SIZE - 1] >= acc_magnitude_max * PEAK_THRESHOLD) {
peak_detected = 1;
acc_magnitude_max = -INFINITY;
}
} else {
// 步数峰值检测到之后,等待加速度模回落到一定程度
if (acc_magnitude_filtered < 0.5 * acc_magnitude_max) {
// 步数加一
step_count++;
peak_detected = 0;
acc_magnitude_max = -INFINITY;
}
}
// 输出步数
printf("Step count: %d\n", step_count);
}
return 0;
}
```
该算法的基本思路是,首先通过低通滤波器去除高频噪声,然后计算加速度模,接着利用滑动窗口和阈值检测找出步数峰值,最后在步数峰值检测到之后等待加速度模回落到一定程度,就可以将步数计数器加一。需要注意的是,该算法仅供参考,实际应用中还需要根据具体情况进行调整和优化。
阅读全文
相关推荐
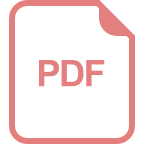
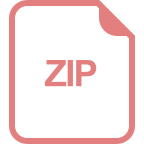

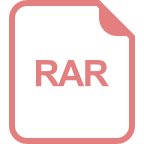
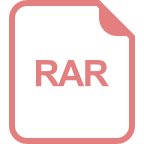
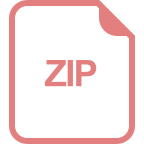
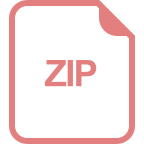
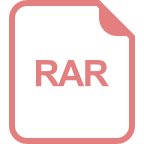
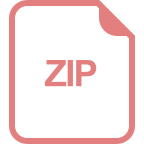
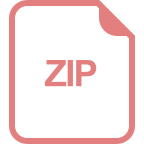
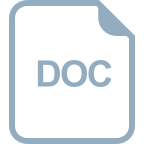
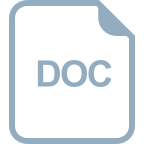
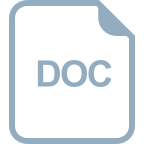
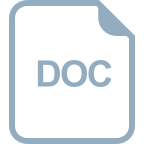
