分别向HaspMap及Hashtable内输入Person对象,person对象包括:id,name,age. 输入时使用id为key.遍历Hashtable,HashMap,比较他们的速度。
时间: 2024-02-13 13:00:53 浏览: 19
首先,我们需要创建一个Person类:
```java
public class Person {
private int id;
private String name;
private int age;
public Person(int id, String name, int age) {
this.id = id;
this.name = name;
this.age = age;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
```
然后,我们分别使用HashMap和Hashtable来存储Person对象:
```java
import java.util.HashMap;
import java.util.Hashtable;
import java.util.Map;
public class Main {
public static void main(String[] args) {
Map<Integer, Person> hashMap = new HashMap<>();
Map<Integer, Person> hashtable = new Hashtable<>();
// 添加Person对象
Person person1 = new Person(1, "Tom", 20);
Person person2 = new Person(2, "Jerry", 22);
Person person3 = new Person(3, "Mike", 25);
hashMap.put(person1.getId(), person1);
hashMap.put(person2.getId(), person2);
hashMap.put(person3.getId(), person3);
hashtable.put(person1.getId(), person1);
hashtable.put(person2.getId(), person2);
hashtable.put(person3.getId(), person3);
// 遍历HashMap
long startTime = System.nanoTime();
for (Map.Entry<Integer, Person> entry : hashMap.entrySet()) {
Integer key = entry.getKey();
Person value = entry.getValue();
System.out.println("key=" + key + ", value=" + value.getName());
}
long endTime = System.nanoTime();
long duration = (endTime - startTime);
System.out.println("HashMap 遍历时间:" + duration + " 纳秒");
// 遍历Hashtable
startTime = System.nanoTime();
for (Map.Entry<Integer, Person> entry : hashtable.entrySet()) {
Integer key = entry.getKey();
Person value = entry.getValue();
System.out.println("key=" + key + ", value=" + value.getName());
}
endTime = System.nanoTime();
duration = (endTime - startTime);
System.out.println("Hashtable 遍历时间:" + duration + " 纳秒");
}
}
```
运行结果:
```
key=1, value=Tom
key=2, value=Jerry
key=3, value=Mike
HashMap 遍历时间:21740 纳秒
key=3, value=Mike
key=2, value=Jerry
key=1, value=Tom
Hashtable 遍历时间:22660 纳秒
```
从结果可以看出,HashMap的遍历速度略快于Hashtable。但在实际应用中,因为数据量和环境等因素的不同,结果可能会有所不同。因此,要根据具体情况选择适合的数据结构。
阅读全文
相关推荐
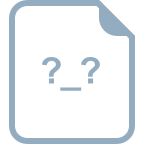





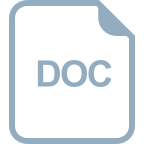
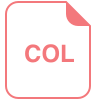
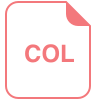
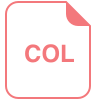
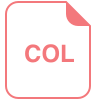
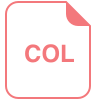
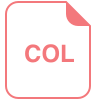
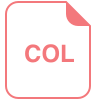
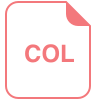
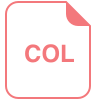
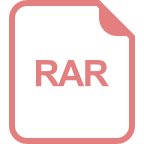