统计字符串中每个元素出现的次数以及有多少个不同的字符C语言
时间: 2024-11-04 09:10:22 浏览: 29
在C语言中,统计字符串中每个元素出现的次数以及计算不同字符的数量可以使用数组、哈希表(如`std::unordered_map`),或者遍历整个字符串并逐个检查字符。以下是使用C标准库实现的一个简单示例:
```c
#include <stdio.h>
#include <ctype.h>
#define MAX_CHAR 128 // 假设ASCII字符集
// 创建一个大小为MAX_CHAR的数组来存储字符计数
int char_count[MAX_CHAR] = {0};
void count_chars(const char *str) {
while (*str) {
if (isalpha(*str)) { // 只考虑字母
char_count[*str - 'a']++; // 字符减去'a'或'A'得到索引
}
str++;
}
}
int main() {
char input[100];
printf("Enter a string: ");
fgets(input, sizeof(input), stdin);
count_chars(input); // 对输入字符串进行计数
int unique_chars = 0;
for (int i = 0; i < MAX_CHAR; i++) {
if (char_count[i] > 0) {
unique_chars++;
printf("'%c': %d\n", i + 'a', char_count[i]); // 输出字符及其计数
}
}
printf("\nTotal unique characters: %d\n", unique_chars);
return 0;
}
```
这个程序首先读取用户输入的字符串,然后遍历该字符串并更新字符计数数组。最后,它找出非零计数的元素,即不同的字符,并输出它们以及各自的频率。
阅读全文
相关推荐
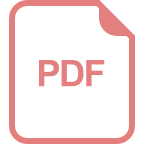
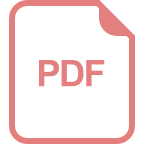
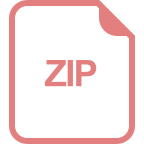















