Yolo实例分割坐标和coco实例分割坐标间转换 python
时间: 2024-01-25 18:04:37 浏览: 186
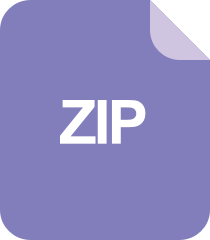
yolo-coco转换器
在Yolo格式中,每个目标都用一个包含其类别、中心点、宽度和高度的矩形来表示。在COCO格式中,每个目标都用一个包含其所有像素的掩码来表示。因此,将Yolo格式转换为COCO格式需要通过计算目标的像素掩码来实现。
以下是将Yolo格式转换为COCO格式的Python代码示例:
```
import numpy as np
import cv2
# Yolo格式坐标转换为COCO格式坐标
def yolo2coco(bbox, img_width, img_height):
x, y, w, h = bbox
left = int((x - w / 2) * img_width)
right = int((x + w / 2) * img_width)
top = int((y - h / 2) * img_height)
bottom = int((y + h / 2) * img_height)
return [left, top, right, bottom]
# COCO格式坐标转换为Yolo格式坐标
def coco2yolo(bbox, img_width, img_height):
left, top, right, bottom = bbox
x = (left + right) / 2 / img_width
y = (top + bottom) / 2 / img_height
w = (right - left) / img_width
h = (bottom - top) / img_height
return [x, y, w, h]
# 读取Yolo格式标注文件
def read_yolo_annotation(annotation_file):
with open(annotation_file, 'r') as f:
lines = f.readlines()
annotations = []
for line in lines:
line = line.strip()
if not line:
continue
parts = line.split()
class_id = int(parts[0])
bbox = [float(x) for x in parts[1:]]
annotations.append((class_id, bbox))
return annotations
# 将Yolo格式标注转换为COCO格式标注
def yolo2coco_annotation(yolo_annotation, img_width, img_height):
coco_annotation = []
for class_id, bbox in yolo_annotation:
coco_bbox = yolo2coco(bbox, img_width, img_height)
mask = np.zeros((img_height, img_width), dtype=np.uint8)
cv2.rectangle(mask, (coco_bbox[0], coco_bbox[1]), (coco_bbox[2], coco_bbox[3]), 1, thickness=-1)
coco_annotation.append({'category_id': class_id, 'bbox': coco_bbox, 'segmentation': mask})
return coco_annotation
# 将COCO格式标注转换为Yolo格式标注
def coco2yolo_annotation(coco_annotation, img_width, img_height):
yolo_annotation = []
for ann in coco_annotation:
class_id = ann['category_id']
coco_bbox = ann['bbox']
yolo_bbox = coco2yolo(coco_bbox, img_width, img_height)
yolo_annotation.append((class_id, yolo_bbox))
return yolo_annotation
```
其中,`yolo2coco`函数将Yolo格式的坐标转换为COCO格式的坐标,`coco2yolo`函数将COCO格式的坐标转换为Yolo格式的坐标。`read_yolo_annotation`函数从Yolo格式的标注文件中读取标注信息,`yolo2coco_annotation`函数将Yolo格式的标注转换为COCO格式的标注,`coco2yolo_annotation`函数将COCO格式的标注转换为Yolo格式的标注。
阅读全文
相关推荐
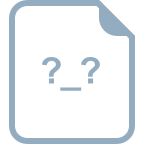
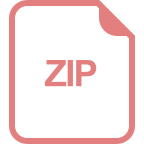
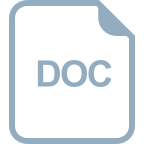
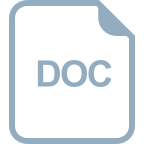
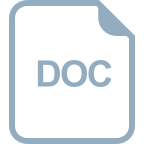
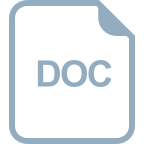
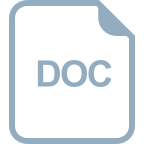









