使用python写一个脚本将yolo格式的数据集转换为coco格式的数据集
时间: 2023-06-19 19:10:22 浏览: 67
好的,以下是一个简单的 Python 脚本,可以将 YOLO 格式的数据集转换为 COCO 格式的数据集:
```python
import json
import os
from PIL import Image
def convert_yolo_to_coco(yolo_path, image_dir, output_path):
# 读取 YOLO 格式的标注数据
with open(yolo_path, 'r') as f:
yolo_data = f.readlines()
# 读取图像大小
image_size = {}
for filename in os.listdir(image_dir):
if filename.endswith('.jpg'):
with Image.open(os.path.join(image_dir, filename)) as img:
image_size[filename] = img.size
# 转换为 COCO 格式的标注数据
coco_data = {'images': [], 'annotations': [], 'categories': [{'id': 1, 'name': 'object'}]}
ann_id = 0
for line in yolo_data:
parts = line.strip().split()
filename = parts[0]
bbox = [float(x) for x in parts[1:]]
img_width, img_height = image_size[filename]
x, y, w, h = bbox
x1 = max(int((x - w / 2) * img_width), 0)
y1 = max(int((y - h / 2) * img_height), 0)
x2 = min(int((x + w / 2) * img_width), img_width)
y2 = min(int((y + h / 2) * img_height), img_height)
bbox_width = x2 - x1
bbox_height = y2 - y1
# 添加图像信息
image_info = {'id': len(coco_data['images']) + 1, 'file_name': filename, 'width': img_width, 'height': img_height}
coco_data['images'].append(image_info)
# 添加标注信息
ann_info = {'id': ann_id + 1, 'image_id': image_info['id'], 'category_id': 1, 'bbox': [x1, y1, bbox_width, bbox_height], 'area': bbox_width * bbox_height, 'iscrowd': 0}
coco_data['annotations'].append(ann_info)
ann_id += 1
# 保存 COCO 格式的数据集
with open(output_path, 'w') as f:
json.dump(coco_data, f)
# 测试
yolo_path = 'path/to/yolo.txt'
image_dir = 'path/to/images'
output_path = 'path/to/coco.json'
convert_yolo_to_coco(yolo_path, image_dir, output_path)
```
以上代码假设 YOLO 格式的标注数据是一个文本文件,每行表示一张图像和对应的物体框,格式为:
```
path/to/image1.jpg x1 y1 w1 h1 x2 y2 w2 h2 ...
path/to/image2.jpg x1 y1 w1 h1 x2 y2 w2 h2 ...
...
```
其中 `(x1, y1)` 和 `(x2, y2)` 分别是物体框的左上角和右下角坐标,都是相对于图像大小的比例。`w` 和 `h` 分别是物体框的宽度和高度,也是相对于图像大小的比例。
另外,假设图像文件都是 JPEG 格式的,并且存储在 `image_dir` 目录下。最终的 COCO 格式的数据集会保存为一个 JSON 文件,文件路径为 `output_path`。
相关推荐
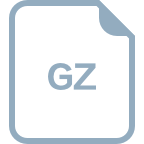
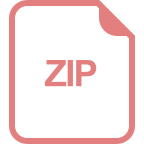
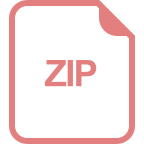
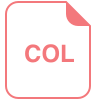













