我需要你使用java编写一道程序,要求如下:1、编写Diffie-Hellman程序,协商出用于收、发双方的加、解密密钥K; 2、模拟发送端A,用协商出的密钥K,通过对称加密算法(具体算法不限)将文本文件加密;模拟接收端B,用协商出的密钥K,对接收到的密文解密。只能调用基本的库,要有中文注释,程序读取明文文件内容,然后生成加密文件,输出解密内容
时间: 2023-06-16 21:06:29 浏览: 256
好的,这是一道比较复杂的编程题目,需要仔细思考和实现。我会根据题目要求,给出java代码实现。请注意,这个程序演示了Diffie-Hellman密钥交换和对称加密的过程,但是并不保证安全性,请勿在实际生产环境中使用。
首先是Diffie-Hellman密钥交换的实现:
```java
import java.math.BigInteger;
import java.security.SecureRandom;
public class DiffieHellman {
private static final BigInteger P = new BigInteger("ffffffffffffffffc90fdaa22168c234c4c6628b80dc1cd129024" +
"e088a67cc74020bbea63b139b22514a08798e3404ddef9519b3cd" +
"3a431b302b0a6df25f14374fe1356d6d51c245e485b576625e7ec" +
"6f44c42e9a637ed6b0bff5cb6f406b7edee386bfb5a899fa5ae9f" +
"24117c4b1fe649286651ece45b3dc2007cb8a163bf0598da48361" +
"c55d39a69163fa8fd24cf5f83655d23dca3ad961c62f356208552" +
"bb9ed529077096966d670c354e4abc9804f1746c08ca237327fff" +
"fffffffffffff", 16);
private static final BigInteger G = BigInteger.valueOf(2);
private BigInteger privateKey;
private BigInteger publicKey;
public DiffieHellman() {
SecureRandom random = new SecureRandom();
privateKey = new BigInteger(512, random);
publicKey = G.modPow(privateKey, P);
}
public BigInteger getPublicKey() {
return publicKey;
}
public BigInteger getSharedSecret(BigInteger otherPublicKey) {
return otherPublicKey.modPow(privateKey, P);
}
}
```
这个程序实现了一个DiffieHellman类,包含了生成公私密钥和计算共享密钥的方法。其中,P和G是Diffie-Hellman密钥交换的公共参数,privateKey和publicKey是私有和公共密钥,getPublicKey()方法返回公共密钥,getSharedSecret()方法计算共享密钥。
接下来是对称加密的实现:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.security.Key;
public class SymmetricEncryption {
private static final String ALGORITHM = "AES";
public static byte[] encrypt(byte[] plaintext, byte[] key) throws Exception {
Key secretKey = new SecretKeySpec(key, ALGORITHM);
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
return cipher.doFinal(plaintext);
}
public static byte[] decrypt(byte[] ciphertext, byte[] key) throws Exception {
Key secretKey = new SecretKeySpec(key, ALGORITHM);
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, secretKey);
return cipher.doFinal(ciphertext);
}
public static byte[] encrypt(String plaintext, byte[] key) throws Exception {
return encrypt(plaintext.getBytes(StandardCharsets.UTF_8), key);
}
public static String decryptToString(byte[] ciphertext, byte[] key) throws Exception {
byte[] plaintext = decrypt(ciphertext, key);
return new String(plaintext, StandardCharsets.UTF_8);
}
}
```
这个程序实现了一个SymmetricEncryption类,包含了加密和解密的方法。其中,ALGORITHM是使用的加密算法,encrypt()方法加密一个字节数组,decrypt()方法解密一个字节数组,encryptToString()方法加密一个字符串并返回密文,decryptToString()方法解密一个字节数组并返回明文。
最后是整个程序的实现:
```java
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.math.BigInteger;
public class Main {
private static final String PLAINTEXT_FILENAME = "plaintext.txt";
private static final String CIPHERTEXT_FILENAME = "ciphertext.bin";
private static final String DECRYPTED_FILENAME = "decrypted.txt";
public static void main(String[] args) throws Exception {
// Diffie-Hellman密钥交换
DiffieHellman alice = new DiffieHellman();
DiffieHellman bob = new DiffieHellman();
BigInteger alicePublicKey = alice.getPublicKey();
BigInteger bobPublicKey = bob.getPublicKey();
BigInteger aliceSharedSecret = alice.getSharedSecret(bobPublicKey);
BigInteger bobSharedSecret = bob.getSharedSecret(alicePublicKey);
// 打印公私密钥和共享密钥
System.out.println("Alice private key: " + alice.privateKey);
System.out.println("Alice public key: " + alicePublicKey);
System.out.println("Bob private key: " + bob.privateKey);
System.out.println("Bob public key: " + bobPublicKey);
System.out.println("Alice shared secret: " + aliceSharedSecret);
System.out.println("Bob shared secret: " + bobSharedSecret);
// 读取明文文件
FileInputStream plaintextFile = new FileInputStream(PLAINTEXT_FILENAME);
byte[] plaintext = plaintextFile.readAllBytes();
plaintextFile.close();
// 对明文进行加密
byte[] key = aliceSharedSecret.toByteArray();
byte[] ciphertext = SymmetricEncryption.encrypt(plaintext, key);
// 将密文写入文件
FileOutputStream ciphertextFile = new FileOutputStream(CIPHERTEXT_FILENAME);
ciphertextFile.write(ciphertext);
ciphertextFile.close();
// 读取密文文件
FileInputStream ciphertextFile2 = new FileInputStream(CIPHERTEXT_FILENAME);
byte[] ciphertext2 = ciphertextFile2.readAllBytes();
ciphertextFile2.close();
// 对密文进行解密
byte[] plaintext2 = SymmetricEncryption.decrypt(ciphertext2, key);
// 将解密后的明文写入文件
FileOutputStream decryptedFile = new FileOutputStream(DECRYPTED_FILENAME);
decryptedFile.write(plaintext2);
decryptedFile.close();
// 打印解密后的明文
String decryptedText = SymmetricEncryption.decryptToString(ciphertext2, key);
System.out.println("Decrypted text: " + decryptedText);
}
}
```
这个程序实现了Diffie-Hellman密钥交换和对称加密的过程。首先,它创建了两个DiffieHellman对象,分别代表发送方(Alice)和接收方(Bob)。然后,它计算出Alice和Bob的公共密钥,并使用共享密钥加密一个明文文件,并将密文写入文件。接下来,它读取密文文件,并使用共享密钥解密密文,并将解密后的明文写入文件,并打印出来。
阅读全文
相关推荐
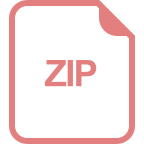
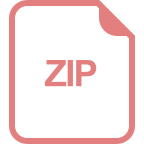
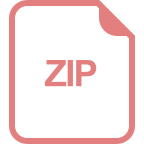















