FileUtils 源码
时间: 2023-12-03 08:53:41 浏览: 183
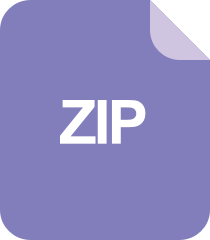
FileUtils:用于处理 Java 文件的辅助类集合
FileUtils 是 Apache Commons IO 库中的一个工具类,提供了一些文件和目录操作的静态方法。以下是其部分源码:
```java
public class FileUtils {
// 省略部分常量定义和构造方法
// ****************** 文件操作 ******************
/**
* 判断文件是否存在
*/
public static boolean isFileExists(File file) {
return file != null && file.exists() && file.isFile();
}
/**
* 创建一个空文件
*/
public static void createEmptyFile(File file) throws IOException {
OutputStream out = new FileOutputStream(file);
out.close();
}
/**
* 复制文件
*/
public static void copyFile(File srcFile, File destFile) throws IOException {
if (!srcFile.exists() || !srcFile.isFile()) {
throw new IllegalArgumentException("Source '" + srcFile + "' does not exist or is not a file");
}
if (destFile.exists() && destFile.isDirectory()) {
throw new IllegalArgumentException("Destination '" + destFile + "' exists but is a directory");
}
try (InputStream in = new FileInputStream(srcFile);
OutputStream out = new FileOutputStream(destFile)) {
byte[] buffer = new byte[4096];
int len;
while ((len = in.read(buffer)) > 0) {
out.write(buffer, 0, len);
}
}
}
/**
* 删除文件或目录
*/
public static void delete(File file) throws IOException {
if (file.isDirectory()) {
cleanDirectory(file);
}
if (!file.delete()) {
throw new IOException("Unable to delete file: " + file);
}
}
// ****************** 目录操作 ******************
/**
* 判断目录是否存在
*/
public static boolean isDirectoryExists(File directory) {
return directory != null && directory.exists() && directory.isDirectory();
}
/**
* 清空目录,但不删除目录本身
*/
public static void cleanDirectory(File directory) throws IOException {
if (!directory.exists()) {
throw new IllegalArgumentException(directory + " does not exist");
}
if (!directory.isDirectory()) {
throw new IllegalArgumentException(directory + " is not a directory");
}
File[] files = directory.listFiles();
if (files == null) { // null if security restricted
throw new IOException("Failed to list contents of " + directory);
}
for (File file : files) {
delete(file);
}
}
/**
* 创建目录,包括其父目录
*/
public static void mkdirs(File directory) throws IOException {
if (!directory.mkdirs() && !directory.isDirectory()) {
throw new IOException("Directory '" + directory + "' could not be created");
}
}
// 省略部分其他方法
}
```
可以看到,FileUtils 提供了一系列静态方法,用于判断文件或目录是否存在、创建文件或目录、复制文件、删除文件或目录等操作。这些方法都是基于 Java 的 I/O 流实现的。
阅读全文
相关推荐
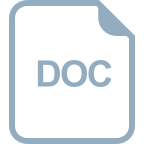
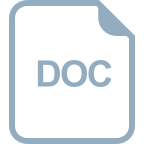
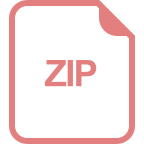
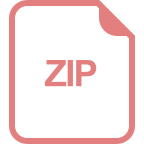
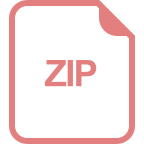
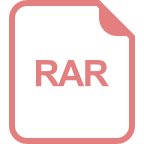
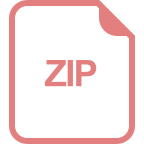
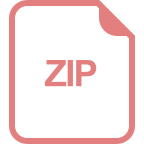
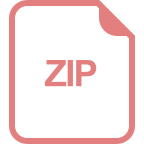
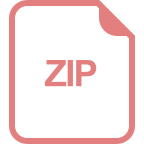
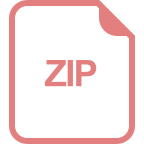
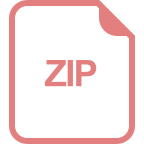
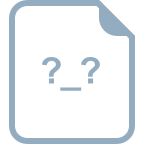
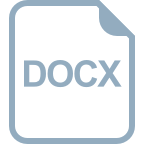
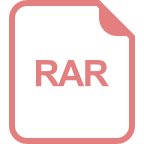
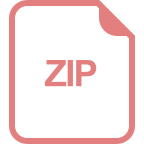
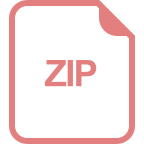