解释这段代码:node* reverseList(node* head) { node* newHead = NULL; node* cur = head; while (cur) { node* next = cur->next; cur->next = newHead; newHead = cur; cur = next; } return newHead; }
时间: 2023-06-02 21:06:57 浏览: 37
这段代码是一个反转链表的函数。传入一个链表的头结点,函数会将链表反转并返回反转后的头结点。具体实现是通过一个while循环,遍历链表中的每个结点,并将当前结点的next指针指向上一个结点,同时更新newHead和cur指针的位置,直到遍历完整个链表。最后返回反转后的头结点即可。
相关问题
struct ListNode* swapPairs(struct ListNode* head){ struct ListNode *current=head->next; struct ListNode *Node=current; current->next=head; current=current->next; while(head!=NULL) { head=head->next->next; current=head->next; current->next=head; current=current->next; } return Node; }优化其代码,减少其运行时间,给出代码
要优化这段代码并减少运行时间,可以尝试使用递归来实现。首先,我们需要考虑终止条件,即当链表为空或者只有一个节点时,无需进行交换,直接返回头节点。然后,我们可以通过递归的方式,交换当前节点和下一个节点的位置,并将当前节点的下一个节点指向递归调用后的结果。最后,返回交换后的头节点。
以下是优化后的代码:
```c
struct ListNode* swapPairs(struct ListNode* head) {
if (head == NULL || head->next == NULL) {
return head;
}
struct ListNode* newHead = head->next;
head->next = swapPairs(newHead->next);
newHead->next = head;
return newHead;
}
```
通过使用递归来交换节点,可以减少代码的复杂性,并且在一次递归调用中实现所有的交换操作。这样可以减少运行时间,并提高代码的可读性。
Node * merge(Node*h1,Node*h2) { Node * p; p=(Node*)malloc(sizeof(Node)); while(h1!=NULL&&h2!=NULL) { if(h1->data>=h2->data) { p->next>=h2; h2=h2->next; } else{ p->next=h1; h1=h1->next; } p=p->next; } if(h1=NULL) { p->next=h2; } else p->next=h1; return p; }为什么无法将有序链表合并
这段代码中有一些错误:
1. 在第7行,应该是 `p->next = h1` 或者 `p->next = h2`,而不是 `p->next >= h2`。
2. 在第14行,应该是 `if(h1==NULL)`,而不是 `if(h1=NULL)`。
除此之外,这个函数并没有将两个有序链表合并成一个有序链表,而是创建了一个新的链表并将较小的节点插入其中,这并没有达到合并的目的。正确的合并方式应该是创建一个新的链表,依次将两个链表中较小的节点插入其中,直到一个链表为空,然后将另一个链表的剩余节点插入新链表的末尾。具体来说,可以参考下面的代码:
```
Node * merge(Node *h1, Node *h2) {
Node *p1 = h1, *p2 = h2, *newHead = NULL, *newTail = NULL;
while (p1 != NULL && p2 != NULL) {
if (p1->data <= p2->data) {
if (newHead == NULL) {
newHead = p1;
newTail = p1;
} else {
newTail->next = p1;
newTail = p1;
}
p1 = p1->next;
} else {
if (newHead == NULL) {
newHead = p2;
newTail = p2;
} else {
newTail->next = p2;
newTail = p2;
}
p2 = p2->next;
}
}
if (p1 != NULL) {
if (newHead == NULL) {
newHead = p1;
} else {
newTail->next = p1;
}
} else {
if (newHead == NULL) {
newHead = p2;
} else {
newTail->next = p2;
}
}
return newHead;
}
```
相关推荐
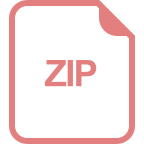
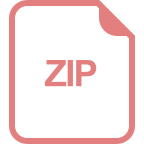
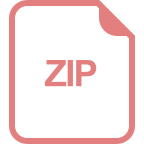













