实时获取chrome中视频网站的帧率 python代码
时间: 2024-06-12 18:07:20 浏览: 16
由于Chrome浏览器的安全限制,Python代码无法直接访问浏览器中的视频帧率。但是,可以使用Chrome DevTools协议来实现这一目标。下面是一个Python代码示例,使用Chrome DevTools协议从Chrome浏览器中获取YouTube视频的帧率:
```python
import json
import requests
# Connect to the Chrome DevTools API
response = requests.get('http://localhost:9222/json/version')
debugger_url = response.json()['webSocketDebuggerUrl']
session = requests.Session()
session.headers.update({'Connection': 'Upgrade'})
response = session.get(debugger_url, stream=True)
websocket_url = response.headers['Location']
websocket = session.get(websocket_url, stream=True)
# Send a message to enable the Page and Performance domains
message = {
'id': 1,
'method': 'Target.setAutoAttach',
'params': {
'autoAttach': True,
'waitForDebuggerOnStart': False,
'flatten': True
}
}
websocket.send(json.dumps(message))
# Send a message to navigate to the YouTube video page
message = {
'id': 2,
'method': 'Page.navigate',
'params': {
'url': 'https://www.youtube.com/watch?v=dQw4w9WgXcQ'
}
}
websocket.send(json.dumps(message))
# Wait for the page to load
while True:
message = json.loads(websocket.recv())
if message['id'] == 2:
break
# Send a message to start tracing performance metrics
message = {
'id': 3,
'method': 'Performance.enable',
'params': {}
}
websocket.send(json.dumps(message))
# Wait for the performance metrics to be collected
while True:
message = json.loads(websocket.recv())
if message['id'] == 3:
break
# Send a message to stop tracing performance metrics
message = {
'id': 4,
'method': 'Performance.disable',
'params': {}
}
websocket.send(json.dumps(message))
# Extract the frame rate from the performance metrics
metrics = message['result']['metrics']
for metric in metrics:
if metric['name'] == 'VideoFrameRate':
print(metric['value'])
break
```
请注意,这个代码示例假设Chrome浏览器已经启动,并且Remote Debugging已经启用。如果您需要自动化Chrome浏览器的启动和关闭,可以使用Python的selenium库。
相关推荐
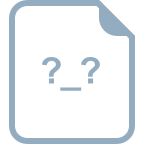
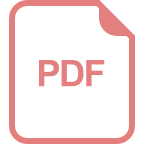
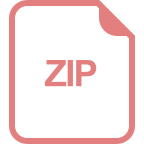







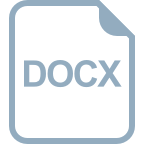