ffmepg 录屏推流 c++示例
时间: 2023-07-05 10:30:51 浏览: 92
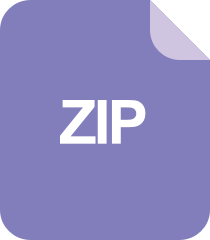
Linux下使用ffmpeg录屏代码
这里提供一个使用 ffmpeg 库进行屏幕录制并推流的 C++ 示例代码:
```c++
#include <iostream>
#include <thread>
#include <chrono>
#include <cstring>
extern "C" {
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/avutil.h>
#include <libavutil/opt.h>
#include <libswscale/swscale.h>
#include <libswresample/swresample.h>
}
#ifdef _WIN32
#include <Windows.h>
#include <d3d9.h>
#pragma comment(lib, "d3d9.lib")
#endif
#define WIDTH 1280
#define HEIGHT 720
#define FPS 30
#define BITRATE 2000000
#define STREAM_URL "rtmp://localhost/live/stream"
int main() {
av_register_all();
avcodec_register_all();
AVFormatContext *format_ctx = nullptr;
AVOutputFormat *output_fmt = nullptr;
AVCodecContext *codec_ctx = nullptr;
AVCodec *codec = nullptr;
AVStream *stream = nullptr;
AVFrame *frame = nullptr;
AVPacket packet;
SwsContext *sws_ctx = nullptr;
// 创建输出格式上下文
avformat_alloc_output_context2(&format_ctx, nullptr, "flv", STREAM_URL);
if (!format_ctx) {
std::cerr << "Could not create output context" << std::endl;
return -1;
}
// 查找视频编码器
codec = avcodec_find_encoder(AV_CODEC_ID_H264);
if (!codec) {
std::cerr << "Could not find encoder" << std::endl;
return -1;
}
// 创建视频流
stream = avformat_new_stream(format_ctx, codec);
if (!stream) {
std::cerr << "Could not create stream" << std::endl;
return -1;
}
codec_ctx = avcodec_alloc_context3(codec);
if (!codec_ctx) {
std::cerr << "Could not allocate codec context" << std::endl;
return -1;
}
// 配置编码器参数
codec_ctx->codec_id = codec->id;
codec_ctx->width = WIDTH;
codec_ctx->height = HEIGHT;
codec_ctx->bit_rate = BITRATE;
codec_ctx->time_base = (AVRational){1, FPS};
codec_ctx->gop_size = 10;
codec_ctx->pix_fmt = AV_PIX_FMT_YUV420P;
// 设置编码器的质量
av_opt_set(codec_ctx->priv_data, "preset", "ultrafast", 0);
av_opt_set(codec_ctx->priv_data, "tune", "zerolatency", 0);
// 打开编码器
if (avcodec_open2(codec_ctx, codec, nullptr) < 0) {
std::cerr << "Could not open codec" << std::endl;
return -1;
}
// 设置视频流参数
avcodec_parameters_from_context(stream->codecpar, codec_ctx);
// 打开输出流
if (avio_open(&format_ctx->pb, STREAM_URL, AVIO_FLAG_WRITE) < 0) {
std::cerr << "Could not open output stream" << std::endl;
return -1;
}
// 写入头信息
avformat_write_header(format_ctx, nullptr);
#ifdef _WIN32
// 初始化 Direct3D
IDirect3D9* d3d = Direct3DCreate9(D3D_SDK_VERSION);
if (!d3d) {
std::cerr << "Could not create Direct3D object" << std::endl;
return -1;
}
// 创建 Direct3D 设备对象
D3DPRESENT_PARAMETERS d3dpp = {0};
d3dpp.Windowed = TRUE;
d3dpp.SwapEffect = D3DSWAPEFFECT_DISCARD;
d3dpp.BackBufferFormat = D3DFMT_UNKNOWN;
d3dpp.hDeviceWindow = GetDesktopWindow();
IDirect3DDevice9* device = nullptr;
if (FAILED(d3d->CreateDevice(D3DADAPTER_DEFAULT, D3DDEVTYPE_HAL, d3dpp.hDeviceWindow,
D3DCREATE_SOFTWARE_VERTEXPROCESSING, &d3dpp, &device))) {
std::cerr << "Could not create Direct3D device" << std::endl;
return -1;
}
// 创建 D3D 表面对象
IDirect3DSurface9* surface = nullptr;
if (FAILED(device->CreateOffscreenPlainSurface(WIDTH, HEIGHT, D3DFMT_X8R8G8B8,
D3DPOOL_SYSTEMMEM, &surface, nullptr))) {
std::cerr << "Could not create surface object" << std::endl;
return -1;
}
#endif
// 分配内存
frame = av_frame_alloc();
av_image_alloc(frame->data, frame->linesize, codec_ctx->width, codec_ctx->height,
codec_ctx->pix_fmt, 32);
// 初始化转换上下文
sws_ctx = sws_getContext(codec_ctx->width, codec_ctx->height, AV_PIX_FMT_BGRA,
codec_ctx->width, codec_ctx->height, codec_ctx->pix_fmt,
SWS_BICUBIC, nullptr, nullptr, nullptr);
while (true) {
#ifdef _WIN32
// 从 D3D 表面对象中获取像素数据
if (FAILED(device->GetFrontBufferData(0, surface))) {
std::cerr << "Could not get front buffer data" << std::endl;
continue;
}
D3DLOCKED_RECT rect;
if (FAILED(surface->LockRect(&rect, nullptr, D3DLOCK_READONLY))) {
std::cerr << "Could not lock surface rect" << std::endl;
continue;
}
// 将像素数据复制到 AVFrame 中
uint8_t *dst_data[4] = {frame->data[0], nullptr, nullptr, nullptr};
int dst_linesize[4] = {frame->linesize[0], 0, 0, 0};
uint8_t *src_data[1] = {(uint8_t*)rect.pBits};
int src_linesize[1] = {rect.Pitch};
sws_scale(sws_ctx, src_data, src_linesize, 0, codec_ctx->height, dst_data, dst_linesize);
surface->UnlockRect();
#else
// TODO: 获取像素数据的代码 (Linux/MacOS)
#endif
// 编码帧
frame->pts = av_gettime();
int ret = avcodec_send_frame(codec_ctx, frame);
if (ret < 0) {
std::cerr << "Error sending frame" << std::endl;
continue;
}
while (ret >= 0) {
ret = avcodec_receive_packet(codec_ctx, &packet);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
break;
} else if (ret < 0) {
std::cerr << "Error receiving packet" << std::endl;
continue;
}
// 发送数据包
av_interleaved_write_frame(format_ctx, &packet);
av_packet_unref(&packet);
}
std::this_thread::sleep_for(std::chrono::milliseconds(1000 / FPS));
}
// 清理资源
av_write_trailer(format_ctx);
avcodec_free_context(&codec_ctx);
avformat_free_context(format_ctx);
av_frame_free(&frame);
sws_freeContext(sws_ctx);
return 0;
}
```
这个示例代码使用了 Direct3D 技术获取屏幕像素数据,因此需要在 Windows 操作系统上运行。如果需要在其他平台上运行,需要修改获取像素数据的代码。
阅读全文
相关推荐
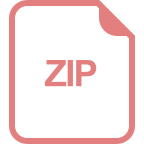
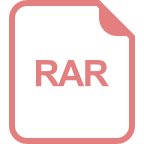















