使用ffmpeg推流C++
时间: 2023-10-10 12:12:47 浏览: 52
以下是使用ffmpeg推流的C代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <pthread.h>
#include <libavformat/avformat.h>
#define STREAM_URL "rtmp://example.com/app/stream"
// 函数声明
void *push_thread(void *arg);
int main(int argc, char *argv[]) {
AVFormatContext *fmt_ctx = NULL;
AVOutputFormat *out_fmt = NULL;
AVStream *video_st = NULL;
AVCodecContext *codec_ctx = NULL;
AVCodec *codec = NULL;
AVDictionary *opt = NULL;
int ret;
pthread_t tid;
// 初始化ffmpeg库
av_register_all();
avformat_network_init();
// 创建输出格式上下文
avformat_alloc_output_context2(&fmt_ctx, NULL, "flv", STREAM_URL);
if (!fmt_ctx) {
fprintf(stderr, "Could not allocate output context\n");
return 1;
}
out_fmt = fmt_ctx->oformat;
// 创建视频流
video_st = avformat_new_stream(fmt_ctx, NULL);
if (!video_st) {
fprintf(stderr, "Could not create video stream\n");
return 1;
}
// 设置编码器参数
codec_ctx = video_st->codec;
codec_ctx->codec_id = out_fmt->video_codec;
codec_ctx->codec_type = AVMEDIA_TYPE_VIDEO;
codec_ctx->pix_fmt = AV_PIX_FMT_YUV420P;
codec_ctx->width = 640;
codec_ctx->height = 480;
codec_ctx->time_base = (AVRational){1, 25};
codec_ctx->bit_rate = 400000;
codec_ctx->gop_size = 10;
// 查找编码器
codec = avcodec_find_encoder(codec_ctx->codec_id);
if (!codec) {
fprintf(stderr, "Could not find encoder\n");
return 1;
}
// 打开编码器
if (avcodec_open2(codec_ctx, codec, &opt) < 0) {
fprintf(stderr, "Could not open encoder\n");
return 1;
}
// 打开输出流
if (!(out_fmt->flags & AVFMT_NOFILE)) {
if (avio_open(&fmt_ctx->pb, STREAM_URL, AVIO_FLAG_WRITE) < 0) {
fprintf(stderr, "Could not open output file '%s'\n", STREAM_URL);
return 1;
}
}
// 写文件头
avformat_write_header(fmt_ctx, &opt);
// 创建推流线程
ret = pthread_create(&tid, NULL, push_thread, fmt_ctx);
if (ret) {
fprintf(stderr, "Could not create push thread\n");
return 1;
}
// 推流循环
while (1) {
// 在这里编码并写入数据
}
// 关闭编码器
avcodec_close(codec_ctx);
// 写文件尾
av_write_trailer(fmt_ctx);
// 释放资源
avformat_free_context(fmt_ctx);
return 0;
}
// 推流线程
void *push_thread(void *arg) {
AVFormatContext *fmt_ctx = (AVFormatContext *)arg;
int ret;
while (1) {
// 在这里读取数据并写入输出流
}
return NULL;
}
```
该示例使用了pthread库来创建一个推流线程,该线程用于读取数据并将其写入输出流。在`while(1)`循环中,可以使用ffmpeg库中的函数进行数据编码和写入操作。需要注意的是,该示例中的`push_thread`函数并没有实现读取和写入操作,需要根据实际情况进行修改。
相关推荐
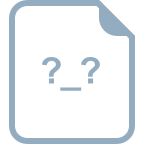














