定义一个房间类( CRoom ),要求拥有的私有数据成员:门牌号( m _ id ),门( m _ pdoor ),窗户( m _ window )。其中,门是门类( CDoor )定义的对象指针成员,窗户是窗户类( CWindow )定义的对象成员。要求:每个类单独定义在两个文件中(. h 和. cpp ),房间类包括:构造函数、析构函数, InstallDoor 成员函数能够实现安装门( new CDoor )的功能。要求窗户类的构造函数必须带参数, CDoor 类具有宽度,高度及修改宽度高度功能◇ CWindow 类具有宽度,高度及修改宽度高度功能。设计好后测试。C++
时间: 2024-01-22 16:17:23 浏览: 79
CRoom.h:
```cpp
#ifndef CROOM_H
#define CROOM_H
#include "CDoor.h"
#include "CWindow.h"
class CRoom {
public:
CRoom(int id);
~CRoom();
void InstallDoor(double width, double height);
private:
int m_id;
CDoor* m_pdoor;
CWindow m_window;
};
#endif
```
CRoom.cpp:
```cpp
#include "CRoom.h"
CRoom::CRoom(int id) {
m_id = id;
m_pdoor = nullptr;
}
CRoom::~CRoom() {
if (m_pdoor != nullptr) {
delete m_pdoor;
}
}
void CRoom::InstallDoor(double width, double height) {
if (m_pdoor != nullptr) {
delete m_pdoor;
}
m_pdoor = new CDoor(width, height);
}
```
CDoor.h:
```cpp
#ifndef CDOOR_H
#define CDOOR_H
class CDoor {
public:
CDoor(double width, double height);
~CDoor();
void SetWidth(double width);
void SetHeight(double height);
private:
double m_width;
double m_height;
};
#endif
```
CDoor.cpp:
```cpp
#include "CDoor.h"
CDoor::CDoor(double width, double height) {
m_width = width;
m_height = height;
}
CDoor::~CDoor() {}
void CDoor::SetWidth(double width) {
m_width = width;
}
void CDoor::SetHeight(double height) {
m_height = height;
}
```
CWindow.h:
```cpp
#ifndef CWINDOW_H
#define CWINDOW_H
class CWindow {
public:
CWindow(double width, double height);
~CWindow();
void SetWidth(double width);
void SetHeight(double height);
private:
double m_width;
double m_height;
};
#endif
```
CWindow.cpp:
```cpp
#include "CWindow.h"
CWindow::CWindow(double width, double height) {
m_width = width;
m_height = height;
}
CWindow::~CWindow() {}
void CWindow::SetWidth(double width) {
m_width = width;
}
void CWindow::SetHeight(double height) {
m_height = height;
}
```
测试代码:
```cpp
#include <iostream>
#include "CRoom.h"
using namespace std;
int main() {
CRoom room1(101);
room1.InstallDoor(1.2, 2.1);
cout << "Door width: " << room1.m_pdoor->GetWidth() << ", height: " << room1.m_pdoor->GetHeight() << endl;
room1.m_pdoor->SetWidth(1.5);
room1.m_pdoor->SetHeight(2.3);
cout << "Door width: " << room1.m_pdoor->GetWidth() << ", height: " << room1.m_pdoor->GetHeight() << endl;
return 0;
}
```
阅读全文
相关推荐
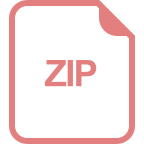
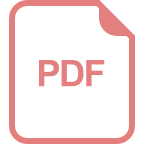
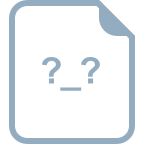





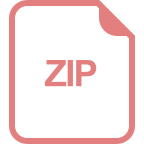
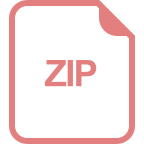
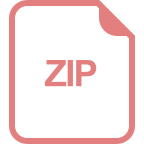
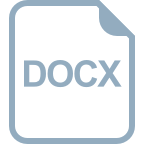
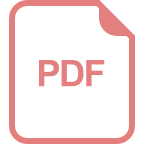
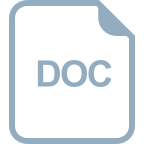
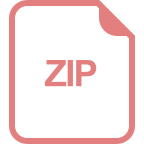